Breaking the Tech Debt Avoidance Loop: Why Python Developers Get Stuck
Key Takeaways
- Technical debt compounds like financial debt, becoming more costly over time
- The avoidance loop starts with small decisions that grow into significant problems
- Python-specific patterns make tech debt particularly challenging
- Breaking the loop requires deliberate strategies and cultural shifts
If you're a Python web developer, you might recognize this scenario: You're racing to ship a feature on deadline. You know there's a cleaner way to implement it, but that would take more time than you have. So you choose the quick solution, promising yourself you'll come back and refactor it later. But "later" never comes. Instead, another urgent feature takes priority, and the cycle continues.
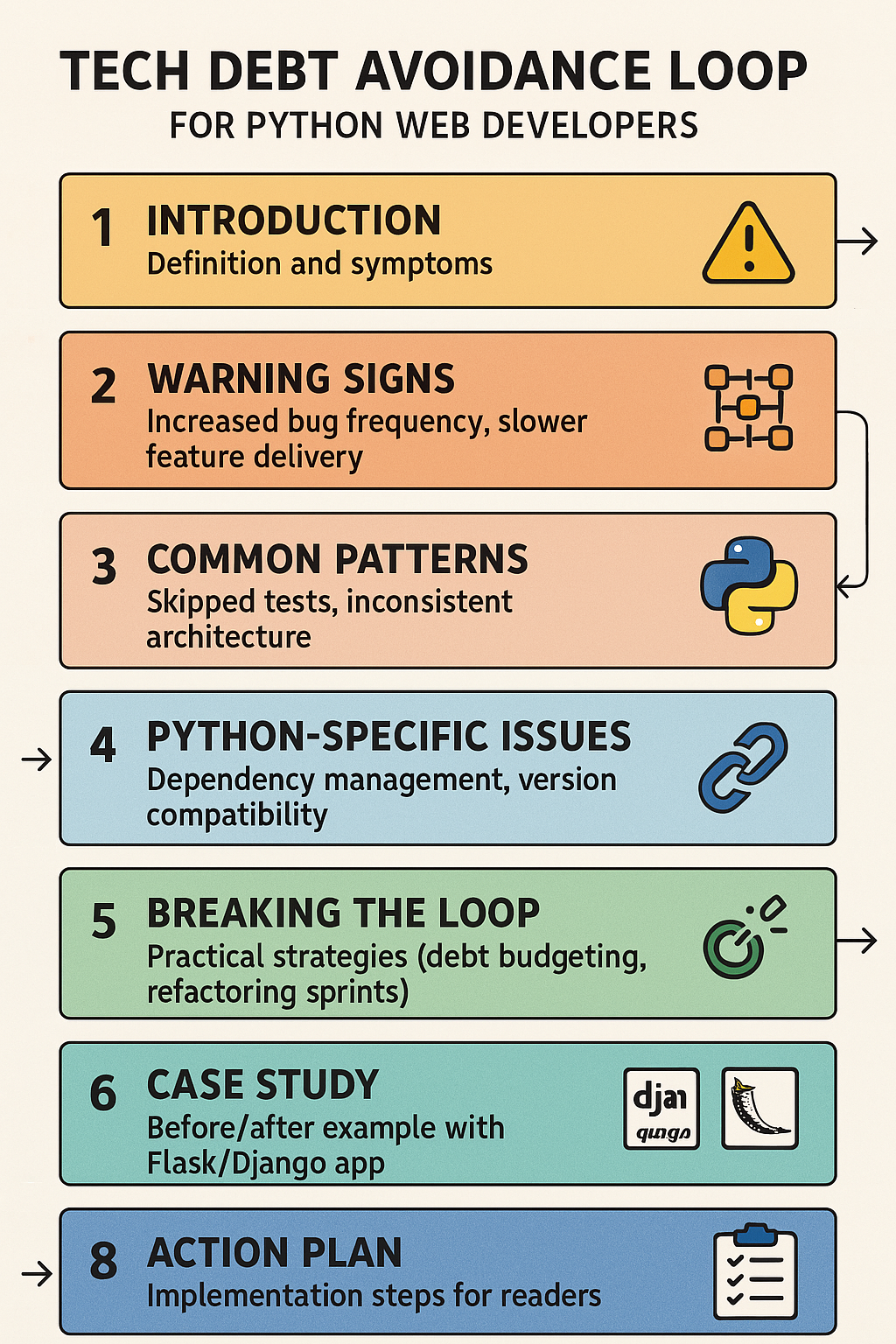
This is the Tech Debt Avoidance Loop - a self-reinforcing cycle where postponing maintenance and improvements leads to increasing technical constraints, which in turn make future work more difficult and time-consuming, further reducing the time available for addressing technical debt.
Warning Signs: You're Stuck in the Loop
The tech debt avoidance loop doesn't announce itself with flashing lights. Instead, it creeps in subtly through these warning signs:
- Velocity Slowdown: Features that once took days now take weeks
- Rising Bug Count: More bugs appearing in seemingly unrelated parts of the codebase
- Developer Frustration: Team members complaining about working with certain parts of the code
- Knowledge Silos: Only one or two developers understand critical components
- Estimate Inflation: Estimates for new work keep increasing
- Resistance to Change: Small changes require touching many files
The Home Maintenance Analogy
To understand tech debt, consider how it parallels home maintenance. Like ignoring a small roof leak that eventually damages walls, floors, and foundation, tech debt compounds silently over time.
Home Issue | Tech Debt Equivalent | Hidden Cost |
---|---|---|
Increased utility bills | Higher server/computing costs | Operational expenses rise while value stays flat |
Declining property value | Decreasing developer productivity | Same team delivers less value over time |
Insurance premium increases | Higher risk management costs | More monitoring, alerts, and incident response needed |
Longer repair times | Extended debugging sessions | Simple fixes take hours instead of minutes |
Specialist contractors needed | Expensive consultants required | External expertise needed at premium rates |
Home becomes unsellable | Codebase becomes unmaintainable | New developers refuse to work on the project |
Eventual condemned status | Complete system rewrite needed | Entire application must be rebuilt from scratch |
Just as homeowners might delay repairs to focus on visible renovations, developers postpone refactoring to ship features. Both situations lead to exponentially higher costs, emergency repairs, and potentially catastrophic failures when systems finally break.
Python-Specific Tech Debt Patterns
Python's flexibility and dynamic nature make it particularly susceptible to certain types of technical debt:
# 1. Type inconsistency debt
def process_item(item):
# Sometimes item is a dict, sometimes a model object
# Sometimes returns a string, sometimes returns a list
if isinstance(item, dict):
return item.get('name', '')
else:
return [item.name]
# 2. Missing docstring debt
def calculate_total(values, apply_discount=False, customer_tier=None):
# Complex function with no documentation
# What do parameters mean? What does it return?
result = sum(values)
if apply_discount:
if customer_tier == 'gold':
result *= 0.9
elif customer_tier == 'silver':
result *= 0.95
return result
# 3. Circular import debt
# In file_a.py
from file_b import ClassB
class ClassA:
def method(self):
return ClassB()
# In file_b.py
from file_a import ClassA # Circular import!
class ClassB:
def method(self):
return ClassA()
# 4. Magic string/number debt
def handle_status(status_code):
if status_code == 1: # What does 1 mean?
process_pending()
elif status_code == 2: # What does 2 mean?
process_active()
# What about other codes?
# 5. Monolithic function debt
def do_everything():
# 200+ line function that handles authentication,
# data validation, processing, saving, and notification
# Impossible to test or maintain
The Real Cost: A Composite Scenario
Let's examine a composite scenario based on common patterns seen across multiple Python web applications that fell into the tech debt avoidance loop:
Typical SaaS Startup Timeline
- Month 1-6: MVP built quickly with Flask. Small technical shortcuts taken to hit market deadlines.
- Month 7-12: Product gains traction. Team expands from 2 to 6 developers. New features prioritized over refactoring.
- Month 13-18: First major outages occur. New feature development slows as team spends 40% of time on bug fixes.
- Month 19-24: Two senior developers quit, citing "unmaintainable codebase" as a reason. Onboarding new developers takes 3x longer than expected.
- Month 25-30: Company commits to a "quick rewrite" of problematic services, estimated at 3 months.
- Month 31-42: Rewrite continues, now at 10 months. Company loses market position as competitors release new features faster.
Estimated cost: Approximately $1-1.5M in developer salaries spent primarily on rework, plus incalculable opportunity cost of delayed features and lost market position.
Breaking the Loop: Practical Strategies
You can escape the tech debt avoidance loop with these actionable strategies:
1. Make Tech Debt Visible
You can't manage what you can't see. Take these steps to visualize your tech debt:
- Create a dedicated tech debt board in your project management system
- Tag issues with "tech-debt" and track them alongside features
- Use tools like Sonarqube or Pylint to quantify code quality metrics
- Generate visual tech debt reports that managers and executives can understand
# Install key Python tools for tech debt visibility
pip install pylint mypy radon xenon
# Basic code quality check
pylint your_module/
# Type checking with mypy
mypy your_module/
# Code complexity metrics
radon cc your_module/ -a
# Detect functions/classes that are too complex
xenon your_module/ --max-absolute B --max-modules A --max-average A
2. Implement the "Boy Scout Rule"
The Boy Scout Rule states: "Always leave the campground cleaner than you found it." Applied to code, this means making small improvements whenever you touch a file.
# Before: You need to modify this function
def calculate_price(product_id, quantity):
prod = Products.get_by_id(product_id)
if prod != None:
base = prod.price
if quantity > 10:
return base * quantity * 0.9
else:
return base * quantity
return 0
# After: You leave it better than you found it
def calculate_price(product_id: str, quantity: int) -> float:
"""Calculate the final price for a product order.
Args:
product_id: The unique identifier for the product
quantity: Number of items being ordered
Returns:
The final price including bulk discounts
Returns 0 if product not found
"""
product = Products.get_by_id(product_id)
if product is None:
return 0.0
base_price = product.price
# Apply 10% discount for bulk orders
discount = 0.9 if quantity > 10 else 1.0
return base_price * quantity * discount
3. Budget for Tech Debt
Formalize tech debt work by allocating time specifically for it:
- Dedicate 20% of each sprint to tech debt reduction
- Schedule regular "refactoring sprints" (1 week every 6-8 weeks)
- Include tech debt reduction in project estimates
- Treat large tech debt items as "features" with their own business case
4. Automate Quality Enforcement
Prevent new tech debt by automating quality checks:
# .pre-commit-config.yaml
repos:
- repo: https://github.com/pre-commit/pre-commit-hooks
rev: v4.4.0
hooks:
- id: trailing-whitespace
- id: end-of-file-fixer
- id: check-yaml
- id: check-added-large-files
- repo: https://github.com/pycqa/isort
rev: 5.12.0
hooks:
- id: isort
args: ["--profile", "black"]
- repo: https://github.com/psf/black
rev: 23.1.0
hooks:
- id: black
language_version: python3.10
- repo: https://github.com/pycqa/flake8
rev: 6.0.0
hooks:
- id: flake8
additional_dependencies: [flake8-docstrings]
- repo: https://github.com/pre-commit/mirrors-mypy
rev: v1.0.1
hooks:
- id: mypy
additional_dependencies: [types-requests]
5. Create a Tech Debt Roadmap
Not all tech debt is created equal. Prioritize with a roadmap:
- Critical: Issues causing production incidents or data corruption
- High: Problems slowing development or increasing bug rates
- Medium: Issues that make code hard to understand or test
- Low: Style issues and minor improvements
Hypothetical Example: Breaking the Loop
Let's look at a hypothetical Flask application that demonstrates breaking out of the tech debt avoidance loop:
# app.py - 4,500 lines of code
from flask import Flask, request, jsonify, render_template
import sqlite3
# 30+ more imports
app = Flask(__name__)
@app.route('/users', methods=['GET'])
def get_users():
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
users = cursor.execute("SELECT * FROM users").fetchall()
conn.close()
return jsonify(users)
@app.route('/users', methods=['POST'])
def create_user():
data = request.json
# No validation
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
cursor.execute(
"INSERT INTO users (name, email) VALUES (?, ?)",
(data['name'], data['email'])
)
conn.commit()
conn.close()
return jsonify({"status": "success"})
# 50+ more routes defined similarly
# app.py - Now just 100 lines
from flask import Flask
from views import register_blueprints
from extensions import db
from config import config_by_name
def create_app(config_name="development"):
app = Flask(__name__)
app.config.from_object(config_by_name[config_name])
# Initialize extensions
db.init_app(app)
# Register blueprints
register_blueprints(app)
return app
# models/user.py
from extensions import db
from dataclasses import dataclass
@dataclass
class User(db.Model):
id: int
name: str
email: str
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(80), nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
# views/user.py
from flask import Blueprint, request, jsonify
from models.user import User
from extensions import db
from schemas.user import UserSchema
user_bp = Blueprint('user', __name__)
user_schema = UserSchema()
@user_bp.route('/users', methods=['GET'])
def get_users():
users = User.query.all()
return jsonify(user_schema.dump(users, many=True))
@user_bp.route('/users', methods=['POST'])
def create_user():
data = user_schema.load(request.json)
new_user = User(**data)
db.session.add(new_user)
db.session.commit()
return jsonify(user_schema.dump(new_user))
In this hypothetical scenario, a team incrementally refactored their monolithic Flask application over three months by:
- Creating a proper project structure with blueprints
- Introducing SQLAlchemy for ORM and migration management
- Adding Marshmallow for validation and serialization
- Implementing proper testing with pytest
- Setting up CI/CD with quality gates
The hypothetical result? Development velocity increased by 60%, onboarding time dropped from weeks to days, and production incidents decreased by 75%.
Action Plan: Your Next Steps
Ready to break your tech debt avoidance loop? Here's your action plan:
Tech Debt Escape Plan
- This week: Run code quality tools on your project and create an inventory of tech debt
- Next week: Implement automated quality checks in your CI/CD pipeline
- Next sprint: Schedule 1-2 days for tackling high-priority tech debt items
- Next month: Create a tech debt roadmap with priorities and estimates
- Next quarter: Schedule a dedicated refactoring sprint
Conclusion: The Virtuous Cycle
Breaking the tech debt avoidance loop isn't just about avoiding problems—it's about creating a virtuous cycle where quality code enables faster development, which in turn allows for more investment in quality.
By making tech debt visible, following the Boy Scout Rule, budgeting for improvements, automating quality enforcement, and creating a tech debt roadmap, you'll transform tech debt from a hidden constraint into a manageable aspect of software development.
Remember: The best time to address tech debt was when you created it. The second best time is now.