Evolution of Testing & Monitoring in Web Frameworks
Testing and monitoring are integral to the development and maintenance of web applications, yet they often remain afterthoughts in many web frameworks. This article examines the evolution of testing and monitoring capabilities in web frameworks, highlighting the persistent gaps between development-time testing and production monitoring, and exploring how these capabilities have either evolved or stagnated over time.
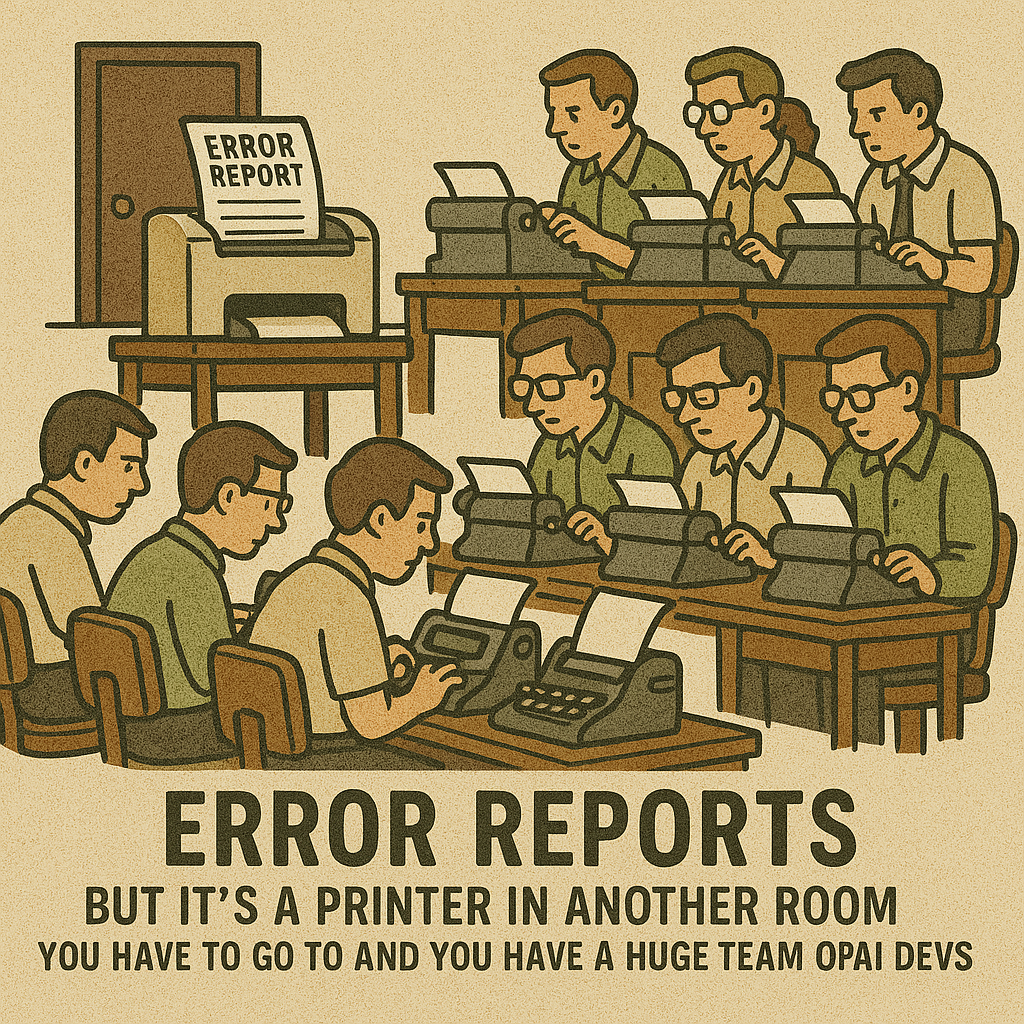
The evolution of testing and monitoring approaches across web framework generations
The Development-Production Disconnect
One of the most persistent challenges in web development is the disconnect between development environments and production realities. Despite decades of framework evolution, this gap remains surprisingly wide:
Development Environment
- Controlled, local environments
- Single-user testing scenarios
- Predictable network conditions
- Immediate error visibility
- Detailed error messages
- Availability of debugging tools
- Test databases with limited data
Production Reality
- Distributed, complex environments
- Concurrent user interactions
- Variable network conditions
- Hidden errors users don't report
- Sanitized error messages (for security)
- Limited debugging capabilities
- Large databases with real user data
This disconnect means applications that work perfectly in development can fail in unexpected ways in production. Surprisingly, most web frameworks still treat production monitoring as an "add-on" rather than an integral part of the framework itself.
The Evolution of Testing in Web Frameworks
Early Days: Manual Testing (1995-2005)
In the early days of web development, testing was primarily manual and ad-hoc:
- Developers would click through pages to verify functionality
- Browser compatibility testing involved running multiple browsers
- Form submissions were tested manually with different inputs
- Errors were discovered mainly through user reports
- Test environments were often simply copies of production
Integrated Testing Frameworks (2005-2015)
The rise of MVC frameworks brought more sophisticated testing approaches:
from django.test import TestCase
from django.urls import reverse
from .models import Product
class ProductTests(TestCase):
def setUp(self):
# Create test data
Product.objects.create(name="Test Product", price="19.99", in_stock=True)
def test_product_list_view(self):
# Test that the product list view returns a 200 status code
response = self.client.get(reverse('product_list'))
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'products/product_list.html')
def test_product_detail_view(self):
# Get the first product from the database
product = Product.objects.first()
# Test that the product detail view for this product returns a 200
response = self.client.get(reverse('product_detail', args=[product.id]))
self.assertEqual(response.status_code, 200)
self.assertContains(response, product.name)
def test_out_of_stock_filter(self):
# Test the filter for out-of-stock products
Product.objects.create(name="Out of Stock Item", price="9.99", in_stock=False)
response = self.client.get(reverse('product_list') + '?in_stock=false')
self.assertContains(response, "Out of Stock Item")
self.assertNotContains(response, "Test Product")
This era saw significant improvements:
- Unit Testing Integration: Frameworks began shipping with built-in test runners
- Test Clients: Simulated HTTP request/response cycles
- Fixtures: Standardized test data management
- Integration with CI Systems: Automated test runs on code changes
- Mocking Libraries: Tools for isolating components during testing
Modern Testing Ecosystem (2015-Present)
The current era has expanded beyond framework-provided testing to include:
import { render, screen, fireEvent } from '@testing-library/react';
import ProductList from './ProductList';
// Mock data
const mockProducts = [
{ id: 1, name: 'Product 1', price: 19.99, inStock: true },
{ id: 2, name: 'Product 2', price: 29.99, inStock: false }
];
// Mock API call
jest.mock('../api', () => ({
fetchProducts: jest.fn().mockResolvedValue(mockProducts)
}));
describe('ProductList Component', () => {
test('renders loading state initially', () => {
render( );
expect(screen.getByText('Loading products...')).toBeInTheDocument();
});
test('renders products when loaded', async () => {
render( );
// Wait for the products to load
const product1 = await screen.findByText('Product 1');
expect(product1).toBeInTheDocument();
expect(screen.getByText('$19.99')).toBeInTheDocument();
});
test('filters out-of-stock products when filter is applied', async () => {
render( );
// Wait for products to load
await screen.findByText('Product 1');
// Click the "In Stock Only" filter
fireEvent.click(screen.getByLabelText('In Stock Only'));
// Product 1 should be visible, Product 2 should be hidden
expect(screen.getByText('Product 1')).toBeInTheDocument();
expect(screen.queryByText('Product 2')).not.toBeInTheDocument();
});
});
Key developments in the modern era include:
- Component Testing: Testing UI components in isolation
- Snapshot Testing: Detecting unintended UI changes
- User Interaction Testing: Simulating clicks, typing, etc.
- End-to-End Testing: Testing complete user journeys
- Visual Regression Testing: Ensuring UI appears as expected
- Headless Browser Testing: Automating browser interactions
- Contract Testing: Verifying API contracts between services
describe('Shopping Cart Functionality', () => {
beforeEach(() => {
// Visit the product page before each test
cy.visit('/products');
// Intercept API calls to ensure consistent test data
cy.intercept('GET', '/api/products', { fixture: 'products.json' });
});
it('allows adding products to cart', () => {
// Find the first product and click its "Add to Cart" button
cy.contains('.product-card', 'Product 1')
.find('.add-to-cart-button')
.click();
// Verify that the cart count increases
cy.get('.cart-count').should('contain', '1');
// Navigate to the cart page
cy.get('.cart-icon').click();
// Verify the product appears in the cart
cy.get('.cart-items').should('contain', 'Product 1');
cy.get('.cart-total').should('contain', '$19.99');
});
it('calculates correct totals when changing quantities', () => {
// Add a product to the cart
cy.contains('.product-card', 'Product 1')
.find('.add-to-cart-button')
.click();
// Go to the cart page
cy.get('.cart-icon').click();
// Change the quantity to 3
cy.get('.quantity-input').clear().type('3');
cy.get('.update-quantity-button').click();
// Verify the total updates correctly
cy.get('.cart-total').should('contain', '$59.97');
});
});
The Declining Role of Traditional Debuggers
An interesting phenomenon in web development has been the gradual decline of traditional debuggers in favor of other approaches:
Traditional Debugging Approach
- Setting breakpoints in code
- Step-by-step execution
- Variable inspection at runtime
- Call stack examination
- Debugger-based watches
Modern Web Development Approach
- Console.log statements
- React/Vue DevTools for component state
- Network tab monitoring
- Hot reloading to test changes quickly
- Time-travel debugging (Redux DevTools)
This shift reflects several realities of modern web development:
- Asynchronous Complexity: Traditional step-through debugging breaks down with promises, callbacks, and event loops
- Component Architecture: Debugging is more about state and props than line-by-line execution
- Multiple Environments: Code often runs in both Node.js and browsers, complicating debugger setup
- Transpilation/Bundling: Source maps often fail to perfectly map to original code
- Distributed Execution: Modern web apps span multiple services and environments
This reality contradicts the common notion that debugger usage is a "best practice" - in modern web development, it's often more effective to use a combination of logging, DevTools, and specialized framework tools than traditional breakpoint debugging.
The Production Monitoring Gap
A significant limitation of most web frameworks is the lack of integrated production monitoring capabilities. This represents one of the largest gaps in the framework responsibility model:
What's Missing from Most Frameworks
- Error Aggregation: Collecting and grouping production errors
- Real User Monitoring: Tracking actual user experience metrics
- Performance Profiling: Identifying slow requests and bottlenecks
- User Journey Tracking: Following users through the application
- Conversion Funnels: Tracking goal completion rates
- Anomaly Detection: Identifying unusual patterns or issues
- Log Correlation: Connecting logs across services
- Synthetic Testing: Regularly verifying critical paths
Instead, these capabilities are typically provided by third-party services like:
- Sentry, Rollbar, or Bugsnag for error tracking
- New Relic, Datadog, or Dynatrace for application performance monitoring
- LogDNA, Papertrail, or Loggly for log management
- FullStory, LogRocket, or Hotjar for session recording
- Google Analytics, Mixpanel, or Amplitude for user analytics
The separation of monitoring from frameworks creates several challenges:
- Integration requires additional configuration and code
- Monitoring often becomes an afterthought rather than a core concern
- Lack of standardization across different monitoring solutions
- No direct feedback loop between production issues and development
The Observability Approach
Modern "observability" combines monitoring, logging, and tracing to provide a comprehensive view of application behavior. Some frameworks are beginning to incorporate aspects of this model:
// Setting up OpenTelemetry in an Express application
const opentelemetry = require('@opentelemetry/sdk-node');
const { getNodeAutoInstrumentations } = require('@opentelemetry/auto-instrumentations-node');
const { OTLPTraceExporter } = require('@opentelemetry/exporter-trace-otlp-http');
const { Resource } = require('@opentelemetry/resources');
const { SemanticResourceAttributes } = require('@opentelemetry/semantic-conventions');
// Configure the SDK with auto-instrumentation
const sdk = new opentelemetry.NodeSDK({
resource: new Resource({
[SemanticResourceAttributes.SERVICE_NAME]: 'my-express-app',
[SemanticResourceAttributes.DEPLOYMENT_ENVIRONMENT]: process.env.NODE_ENV
}),
traceExporter: new OTLPTraceExporter({
url: 'http://otel-collector:4318/v1/traces',
}),
instrumentations: [getNodeAutoInstrumentations()]
});
// Initialize OpenTelemetry
sdk.start();
// Later in your Express app
const express = require('express');
const app = express();
app.get('/products', async (req, res) => {
// This request will be automatically traced
const products = await db.getProducts();
res.json(products);
});
However, even these newer approaches typically require explicit integration rather than being built into the framework core.
Analytics: Beyond Page Views
While Google Analytics has dominated web analytics for years, modern applications require more sophisticated tracking capabilities that are rarely built into frameworks:
Limitations of Traditional Analytics
- Focus on page views rather than user interactions
- Limited ability to track single-page application interactions
- Poor integration with application state and context
- Separate from application code and data
- Increasingly blocked by privacy tools and browsers
- Growing privacy regulation constraints
Modern Product Analytics Needs
- Event-Based Tracking: Detailed user interactions beyond page views
- User Identification: Connecting behaviors to specific users
- Conversion Funnels: Tracking multi-step processes
- Cohort Analysis: Comparing user groups over time
- Session Replay: Visualizing actual user journeys
- Feature Usage Tracking: Understanding which features are used
- A/B Testing Integration: Measuring impact of variations
Modern implementations often use specialized product analytics tools:
import { useEffect } from 'react';
import { useLocation } from 'react-router-dom';
import { useAnalytics } from '../hooks/useAnalytics';
function CheckoutForm({ cartItems, totalAmount }) {
const location = useLocation();
const { trackEvent, trackPageView, identifyUser } = useAnalytics();
// Track page view when the component mounts or route changes
useEffect(() => {
trackPageView({
path: location.pathname,
title: 'Checkout Page',
properties: {
cartItemCount: cartItems.length,
cartValue: totalAmount
}
});
}, [location, trackPageView, cartItems.length, totalAmount]);
// Track specific user actions
const handlePaymentMethodSelect = (method) => {
trackEvent('payment_method_selected', {
method,
cartValue: totalAmount
});
// Continue with payment method selection logic
setSelectedPaymentMethod(method);
};
const handleSubmitOrder = () => {
// Track the conversion event
trackEvent('order_completed', {
orderId: generatedOrderId,
products: cartItems.map(item => ({
id: item.id,
name: item.name,
price: item.price,
quantity: item.quantity
})),
total: totalAmount,
paymentMethod: selectedPaymentMethod,
shippingMethod: selectedShippingMethod
});
// Continue with order submission logic
submitOrder();
};
return (
);
}
This disconnect between web frameworks and analytics leads to several problems:
- Duplicate Logic: Business logic gets replicated in analytics code
- Data Inconsistency: Analytics data may not match application state
- Implementation Overhead: Every interaction needs explicit tracking
- Maintenance Burden: Analytics code needs to be updated with application changes
More sophisticated approaches integrate analytics at the framework level:
// Analytics middleware for Redux
const analyticsMiddleware = (analyticsClient) => store => next => action => {
// First, let the action go through to update state
const result = next(action);
// Then track specific actions of interest
switch (action.type) {
case 'cart/addItem':
analyticsClient.trackEvent('product_added_to_cart', {
productId: action.payload.id,
productName: action.payload.name,
price: action.payload.price,
quantity: action.payload.quantity
});
break;
case 'checkout/complete':
const state = store.getState();
analyticsClient.trackEvent('purchase_completed', {
orderId: action.payload.orderId,
products: state.cart.items,
total: state.cart.total,
currency: state.app.currency
});
break;
case 'user/login':
analyticsClient.identifyUser(action.payload.userId, {
email: action.payload.email,
// Only include non-sensitive user properties
userType: action.payload.userType,
accountCreated: action.payload.createdAt
});
break;
}
return result;
};
// Usage in store configuration
import { createStore, applyMiddleware } from 'redux';
import rootReducer from './reducers';
import analyticsClient from './analytics';
const store = createStore(
rootReducer,
applyMiddleware(analyticsMiddleware(analyticsClient))
);
However, even these more integrated approaches are rarely built into frameworks themselves, requiring additional configuration and custom implementation.
Testing & Monitoring Capabilities Across Framework Generations
Capability | CGI Era (1995-2000) | MVC Frameworks (2000-2010) | JavaScript Frameworks (2010-2020) | Modern Frameworks (2020+) |
---|---|---|---|---|
Unit Testing | Limited, external tools only | Built-in test runners, mocking support | Component testing, snapshot testing | Testing as first-class concern |
End-to-End Testing | Manual only | Basic browser automation | Dedicated testing frameworks (Cypress, Puppeteer) | Integration with testing services, visual testing |
Error Reporting | Server logs only | Framework error pages, basic logging | Third-party error tracking integration | Error boundary components, contextual errors |
Performance Monitoring | None | Basic request timing | Web Vitals support, performance hooks | Real User Monitoring integration points |
Debugging Tools | Print statements | Built-in debug modes | Framework DevTools, time-travel debugging | Hot reloading, state inspection |
User Analytics | Server access logs | GA integration examples | Event-based analytics helpers | Privacy-focused, server-side analytics options |
Logging | Server logs only | Framework logging systems | Structured logging | Context-aware logging, tracing integration |
Security Testing | None | Basic CSRF testing | Security middleware testing | Dependency scanning integration, SAST hooks |
This evolution shows general improvement over time, but also highlights that many modern monitoring and analytics capabilities remain outside the core framework responsibilities.
Emerging Solutions and Future Directions
Several emerging approaches aim to bridge the development-production gap:
Frameworks designed with instrumentation as a first-class concern:
- Built-in OpenTelemetry integration
- Automatic trace context propagation
- Performance metrics emitted by default
- Structured logging with correlation IDs
- Health check endpoints
- Standardized error reporting
Examples beginning to emerge in serverless frameworks and service meshes
Integrating analytics with application state management:
- Analytics middleware for state containers
- Declarative event tracking
- Event schemas with validation
- User consent management
- Server-side analytics for privacy
- Real-time analytics dashboards
Some Next.js applications now implement this pattern with custom middleware
Blending testing and monitoring for continuous validation:
- Canary deployments with automatic testing
- Synthetic transaction monitoring
- A/B test infrastructure
- Feature flag testing frameworks
- Production experiments
- Real-time feedback loops
Companies like Netflix and Google pioneering these approaches
The Ideal Future State
Looking ahead, web frameworks could evolve to include:
- Unified Observability: Built-in tracing, metrics, and logging that work together
- Test-to-Production Continuity: Test assertions that can run in production as monitors
- Integrated Analytics: First-class analytics that understand application context
- Real User Monitoring: Performance and error tracking built into rendering layers
- Declarative User Journeys: Define critical paths that are both tested and monitored
- Debugging Anywhere: Consistent debugging experiences across development and production
- Privacy-First Monitoring: Data collection that respects user consent and regulations
Practical Steps for Developers Today
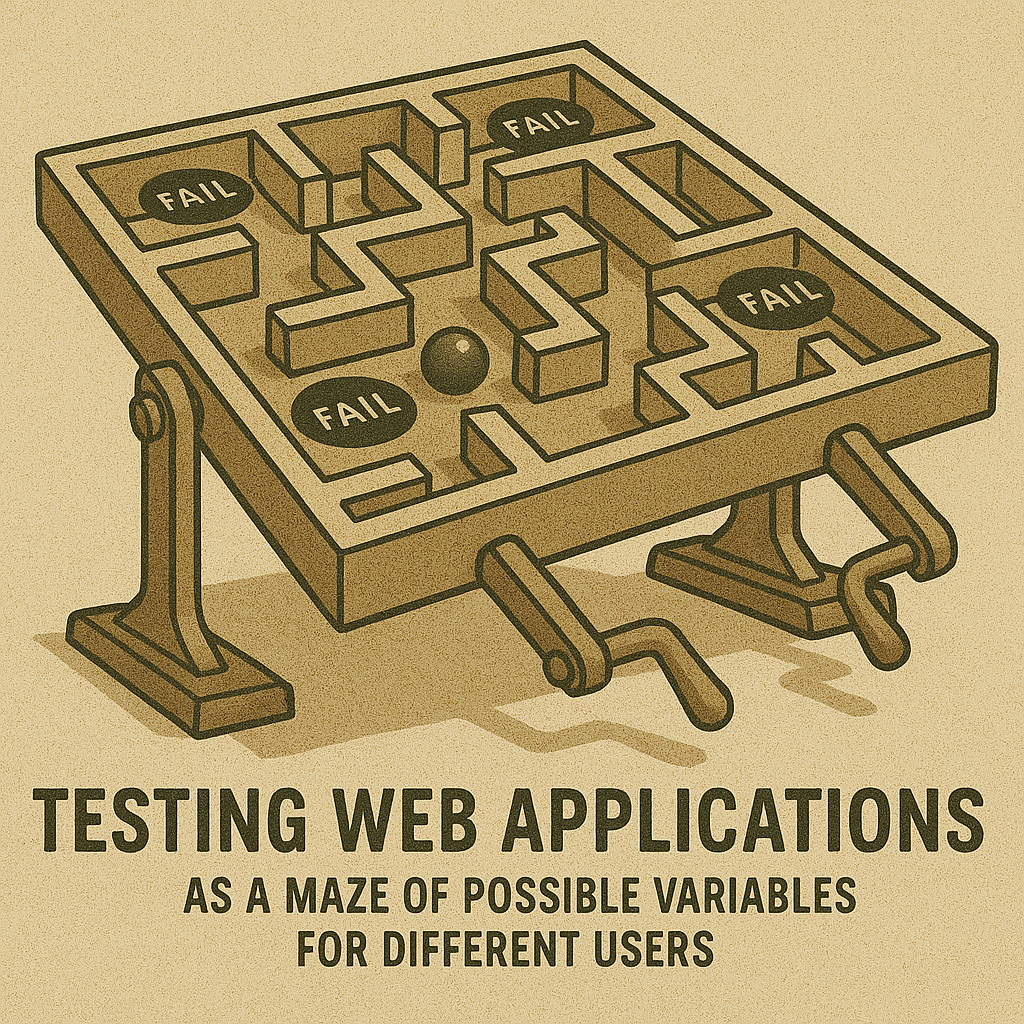
Testing and monitoring workflows across the full development lifecycle
While waiting for frameworks to evolve, developers can take these steps to bridge the gap:
Improving Testing
- Write tests that mirror real user behaviors, not just code coverage
- Include network conditions and error states in end-to-end tests
- Test with realistic data volumes and performance constraints
- Implement visual regression testing for UI components
- Regularly run security and accessibility tests
- Use property-based testing for edge cases
- Test in multiple viewports and browsers
Enhancing Monitoring
- Implement a structured logging strategy with context
- Add OpenTelemetry instrumentation to key services
- Track real user metrics (Core Web Vitals) in production
- Create custom dashboards for business-critical flows
- Set up alerting based on user experience, not just system metrics
- Implement error boundaries with detailed reporting
- Use session replay for understanding user journeys
Integrating Testing and Monitoring
- Reuse test assertions for monitoring health checks
- Implement synthetic monitoring for critical user flows
- Create a unified event schema for testing and analytics
- Build custom middleware to standardize logging and tracing
- Create development tools that replicate production observability
- Use feature flags to control rollout and monitor new features
// Define event schema with TypeScript for type safety
type EventName =
| 'page_view'
| 'button_click'
| 'form_submit'
| 'product_view'
| 'add_to_cart'
| 'checkout_start'
| 'checkout_complete';
interface BaseEventProperties {
timestamp: number;
sessionId: string;
userId?: string;
}
interface PageViewEvent extends BaseEventProperties {
name: 'page_view';
path: string;
referrer?: string;
title?: string;
}
interface ButtonClickEvent extends BaseEventProperties {
name: 'button_click';
buttonId: string;
buttonText: string;
location: string;
}
interface ProductViewEvent extends BaseEventProperties {
name: 'product_view';
productId: string;
productName: string;
price: number;
category: string;
}
// Union type of all possible events
type AppEvent =
| PageViewEvent
| ButtonClickEvent
| ProductViewEvent
// | Other event types...
// Unified tracking function used in both tests and production
const trackEvent = (event: AppEvent) => {
// Add common properties
const eventWithMetadata = {
...event,
environment: process.env.NODE_ENV,
appVersion: APP_VERSION,
timestamp: event.timestamp || Date.now()
};
// In tests, store events for assertions
if (process.env.NODE_ENV === 'test') {
eventStore.push(eventWithMetadata);
return;
}
// In development, log to console
if (process.env.NODE_ENV === 'development') {
console.log('EVENT:', eventWithMetadata);
}
// In production, send to analytics service
if (process.env.NODE_ENV === 'production') {
analyticsService.trackEvent(eventWithMetadata);
// Also send to monitoring service for certain events
if (
event.name === 'checkout_start' ||
event.name === 'checkout_complete'
) {
monitoringService.recordUserJourney(eventWithMetadata);
}
}
};
Conclusion
Testing and monitoring remain somewhat fragmented aspects of web development. While testing has become increasingly integrated into web frameworks, production monitoring and analytics largely remain separate concerns requiring additional integration.
The disconnect between development-time testing and production monitoring represents one of the most significant gaps in the web framework responsibility model. Despite the critical importance of understanding application behavior in production, most frameworks provide limited built-in capabilities for observability, analytics, and continuous validation.
As web applications grow more complex and distributed, this gap becomes increasingly problematic. The future of web frameworks likely includes more unified approaches to testing and monitoring, with observability as a first-class concern rather than an afterthought.
Until then, developers must continue to bridge this gap themselves, creating connections between their testing infrastructure and production monitoring systems to ensure their applications not only work correctly in development but continue to deliver value reliably in production.