Evolution of Internationalization & Localization in Web Frameworks
As the internet evolved from an English-centric medium to a truly global platform, web applications needed to adapt to users speaking different languages and living in different cultures. This article traces the evolution of internationalization (i18n) and localization (l10n) in web frameworks, from rudimentary approaches to sophisticated systems that handle everything from translations to culturally-appropriate formatting.
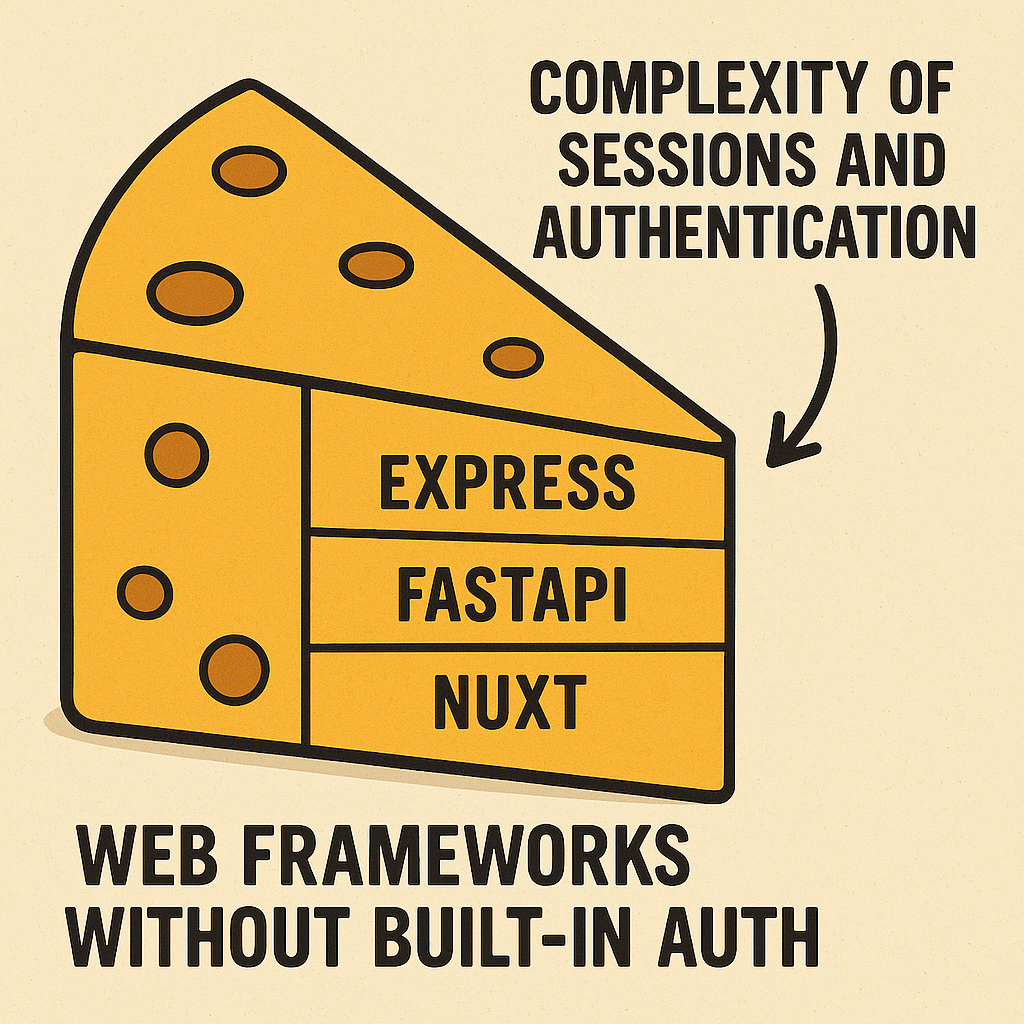
From hardcoded text to sophisticated translation systems: The evolution of multilingual web support
The Early Web: Hardcoded Content (1990s)
In the earliest days of the web, internationalization was rarely a consideration. Web pages were predominantly in English, with the following characteristics:
- Manual Translation: Separate HTML files for each language
- No Standardization: Ad hoc approaches to language selection
- Limited Character Support: ASCII dominance with poor support for non-Latin scripts
- Hardcoded Format: Dates, numbers, and currencies in English/US formats
/
├── index.html # English default
├── en/
│ └── index.html # English content
├── fr/
│ └── index.html # French content
├── de/
│ └── index.html # German content
└── es/
└── index.html # Spanish content
This approach required complete duplication of content for each language, making maintenance extremely difficult. Each change needed to be manually implemented across all language versions, leading to frequent inconsistencies and outdated translations.
Server-Side Templating Era: Basic Separation (Late 1990s - Early 2000s)
As dynamic web applications emerged, early server-side technologies like PHP and ASP began implementing basic translation systems:
<?php
// lang/en.php
$lang = array(
'welcome' => 'Welcome to our website',
'login' => 'Log in',
'register' => 'Register',
'email' => 'Email address',
'password' => 'Password',
'submit' => 'Submit'
);
// lang/fr.php
$lang = array(
'welcome' => 'Bienvenue sur notre site web',
'login' => 'Connexion',
'register' => 'S\'inscrire',
'email' => 'Adresse e-mail',
'password' => 'Mot de passe',
'submit' => 'Envoyer'
);
// index.php
session_start();
$language = isset($_SESSION['lang']) ? $_SESSION['lang'] : 'en';
include_once "lang/$language.php";
?>
<html>
<head><title><?php echo $lang['welcome']; ?></title></head>
<body>
<h1><?php echo $lang['welcome']; ?></h1>
<form>
<label><?php echo $lang['email']; ?></label>
<input type="email" name="email">
<label><?php echo $lang['password']; ?></label>
<input type="password" name="password">
<button type="submit"><?php echo $lang['submit']; ?></button>
</form>
</body>
</html>
Key improvements in this era included:
- Text Separation: Translation strings in separate files
- Language Detection: Basic user language preferences via cookies or sessions
- Language Switching: Manual language selection for users
- Character Encoding: Emergence of UTF-8 support for multiple scripts
However, this approach still had significant limitations:
- No standardized way to handle pluralization rules
- Poor support for right-to-left languages
- No context provided for translators
- Date, number, and currency formatting still largely hardcoded
MVC Framework Era: Standardized Approaches (Mid-2000s)
With the rise of MVC frameworks like Ruby on Rails, Django, and ASP.NET MVC, internationalization became more structured and comprehensive:
# settings.py
LANGUAGE_CODE = 'en-us'
USE_I18N = True
USE_L10N = True
LANGUAGES = [
('en', 'English'),
('fr', 'French'),
('de', 'German'),
('es', 'Spanish'),
]
LOCALE_PATHS = [
os.path.join(BASE_DIR, 'locale'),
]
# models.py
from django.db import models
from django.utils.translation import gettext_lazy as _
class Product(models.Model):
name = models.CharField(_('product name'), max_length=100)
description = models.TextField(_('product description'))
price = models.DecimalField(_('price'), max_digits=10, decimal_places=2)
class Meta:
verbose_name = _('product')
verbose_name_plural = _('products')
# views.py
from django.utils.translation import gettext as _
from django.http import HttpResponse
def welcome(request):
output = _('Welcome to our store!')
return HttpResponse(output)
# templates/base.html
{% load i18n %}
{% trans "My Store" %}
{% trans "Welcome to our store" %}
{% blocktrans with name=user.name %}Hello, {{ name }}!{% endblocktrans %}
{% blocktrans count counter=items_count %}
You have {{ counter }} item in your cart.
{% plural %}
You have {{ counter }} items in your cart.
{% endblocktrans %}
Framework-based internationalization introduced several key advancements:
- Translation Functions: Specialized functions/tags for marking translatable strings
- Automatic Message Extraction: Tools to scan code and templates for translatable strings
- Translation File Formats: Standardized formats like GNU gettext PO files
- Pluralization Support: Rules for handling quantity-based language variations
- Context Notes: Adding context for translators
- Locale Data: Proper date/time, number, and currency formatting
- Translation Management: Tooling for managing translation workflows
Language Detection & Selection Strategies
Over time, various approaches emerged for detecting and selecting the appropriate language for users:
Strategy | Implementation | Pros | Cons |
---|---|---|---|
Browser Accept-Language | Use the browser's language preferences from HTTP headers | Automatic, respects user preferences | Shared devices may use wrong preferences |
GeoIP-based | Detect user's country by IP address and infer language | Works without prior user interaction | Travelers may get incorrect language, privacy concerns |
URL Path Prefix | Use URL paths like /en/, /fr/, /de/ | Explicit, bookmarkable, SEO-friendly | Longer URLs, requires routing configuration |
Subdomain | Use fr.example.com, es.example.com | Clear separation, easier CDN configuration | Requires additional DNS setup, cookie challenges |
Top-level Domain | Use example.fr, example.de, example.es | Strong geographic/cultural association | Expensive, requires multiple domain registrations |
User Preference | Allow users to explicitly select language, store in profile | Most accurate for logged-in users | Requires user action, not helpful for first visit |
Query Parameter | Use ?lang=fr&locale=fr_FR | Simple to implement | Not persistent, poor SEO, less professional |
# settings.py
MIDDLEWARE = [
# ...
'django.middleware.locale.LocaleMiddleware',
# ...
]
# URLs with language prefix
from django.conf.urls.i18n import i18n_patterns
from django.urls import path, include
urlpatterns = [
path('i18n/', include('django.conf.urls.i18n')), # Language switch view
]
# Add URLs that should be translated
urlpatterns += i18n_patterns(
path('', include('myapp.urls')),
prefix_default_language=False, # Don't add prefix for default language
)
Most modern frameworks implement a hierarchical fallback system:
- Explicit user preference (if logged in or stored in cookie/session)
- URL path/domain indicator (if present)
- Browser Accept-Language header
- GeoIP-based guess (if enabled)
- Default application language
Translation File Management
The organization and management of translation files evolved substantially:
# 1. Extract messages from Python code and templates
python manage.py makemessages -l fr
# This creates/updates locale/fr/LC_MESSAGES/django.po file with entries like:
#: models.py:23
msgid "product name"
msgstr "nom du produit"
#: templates/store/product_detail.html:15
msgid "Add to cart"
msgstr "Ajouter au panier"
# 2. Translators edit .po files
# 3. Compile message files for production
python manage.py compilemessages
Translation File Formats
- Gettext PO Files: Text-based, human-readable format (Django, Rails)
- JSON Files: Common in JavaScript frameworks (React-i18next, Vue-i18n)
- YAML Files: Human-readable hierarchical format (Rails)
- XLIFF: XML-based format for exchange between translation tools
- RESX Files: XML resource files (ASP.NET)
Professional Translation Workflow Tools
As applications scaled, dedicated translation management systems emerged:
- Localization Platforms: Lokalise, Phrase, Crowdin, POEditor
- Continuous Integration: Automatic extraction during builds
- Translation Memory: Reuse previously translated strings
- Machine Translation: AI-assisted translation suggestions
- Context Screenshots: Visual context for translators
- GitHub Integration: Pull request workflows for translations
Date, Number, and Currency Formatting
Proper localization extends beyond text translation to formatting conventions:
Format | en-US | fr-FR | de-DE | ja-JP |
---|---|---|---|---|
Short Date | 4/11/2025 | 11/04/2025 | 11.04.2025 | 2025/04/11 |
Long Date | April 11, 2025 | 11 avril 2025 | 11. April 2025 | 2025年4月11日 |
Time | 3:30 PM | 15:30 | 15:30 | 15時30分 |
Format | en-US | fr-FR | de-DE | hi-IN |
---|---|---|---|---|
Large Number | 1,234,567.89 | 1 234 567,89 | 1.234.567,89 | 12,34,567.89 |
Currency | $1,234.56 | 1 234,56 € | 1.234,56 € | ₹ 1,234.56 |
Percentage | 56.7% | 56,7 % | 56,7 % | 56.7% |
{% load l10n i18n %}
{% now "SHORT_DATE_FORMAT" %}
{% now "LONG_DATE_FORMAT" %}
{% now "j F Y" %}
{{ value|localize }}
{{ value|floatformat:2 }}
{{ price|currency:"USD" }}
{% timezone "Europe/Paris" %}
{% now "H:i" %}
{% endtimezone %}
Modern frameworks leverage the Unicode Common Locale Data Repository (CLDR), which provides standardized rules for formatting dates, numbers, and other locale-specific data across nearly 200 languages.
Right-to-Left (RTL) Language Support
Supporting languages like Arabic, Hebrew, and Persian requires special considerations:
- Text Direction: HTML dir attribute and CSS direction property
- CSS Mirroring: Flipping layouts, margins, padding, borders
- Bidirectional Text: Handling mixed LTR/RTL content
- UI Elements: Mirroring navigation, icons, and interactive elements
import styled from 'styled-components';
import { useTranslation } from 'react-i18next';
const Button = styled.button`
margin-inline-start: 10px;
padding: 8px 16px;
${props => props.rtl && `
/* Additional RTL-specific styling if needed */
font-family: 'Arabic Font', sans-serif;
`}
`;
function SubmitButton() {
const { t, i18n } = useTranslation();
const isRTL = i18n.dir() === 'rtl';
return (
);
}
Modern frameworks and CSS now provide better tools for handling RTL layouts:
- CSS Logical Properties: Using start/end instead of left/right
- RTL Libraries: Tools like RTLCSS to automatically flip stylesheets
- Framework Support: Built-in RTL helpers in UI libraries
- Flexbox and Grid: Using modern layout techniques that adapt to direction
The RTL challenge extends beyond just flipping layouts—it requires consideration of:
- Phone number and currency input fields
- Calendar widgets (week starts on different days)
- Iconography (some icons need mirroring, others don't)
- Scroll direction and animation direction
- Text alignment for mixed LTR/RTL content
Modern JavaScript Framework Approaches
Client-side JavaScript frameworks have developed sophisticated i18n libraries:
// i18n.js - Configuration
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
import Backend from 'i18next-http-backend';
import LanguageDetector from 'i18next-browser-languagedetector';
i18n
.use(Backend)
.use(LanguageDetector)
.use(initReactI18next)
.init({
fallbackLng: 'en',
ns: ['common', 'product', 'checkout'],
defaultNS: 'common',
interpolation: {
escapeValue: false
}
});
// Component usage
import { useTranslation, Trans } from 'react-i18next';
function ProductDetail({ product }) {
const { t, i18n } = useTranslation(['product', 'common']);
return (
{/* Basic translation */}
{t('product:title')}
{/* With interpolation */}
{t('price', { value: product.price })}
{/* Complex translation with formatting */}
This product by {{ brand }} offers excellent quality.
{/* Pluralization */}
{t('common:itemCount', { count: product.itemCount })}
{/* Formatted date */}
{new Intl.DateTimeFormat(i18n.language).format(product.releaseDate)}
{/* Formatted currency */}
{new Intl.NumberFormat(i18n.language, {
style: 'currency',
currency: 'USD'
}).format(product.price)}
);
}
Other modern frameworks offer similar capabilities:
Welcome to our store
Hello, {{ user.name }}!
Updated: {{ lastUpdate | date }}
{itemCount, plural,
=0 {No items in cart}
=1 {One item in cart}
other {{{ itemCount }} items in cart}
}
// i18n configuration
import Vue from 'vue';
import VueI18n from 'vue-i18n';
Vue.use(VueI18n);
const i18n = new VueI18n({
locale: 'en',
fallbackLocale: 'en',
messages: {
en: {
welcome: 'Welcome',
items: 'no items | one item | {count} items'
},
fr: {
welcome: 'Bienvenue',
items: 'aucun article | un article | {count} articles'
}
}
});
// Component usage
{{ $t('welcome') }}
{{ $tc('items', cartCount) }}
{{ $d(new Date(), 'short') }}
{{ $n(price, 'currency') }}
Modern front-end internationalization solutions offer several advantages:
- Dynamic Language Switching: Change language without page reload
- Lazy Loading: Load translation files only when needed
- Integration with Formatters: Using Intl API for date/number formatting
- Component-Level Translations: Scoped translation namespaces
- Translation Management: Integration with various backend services
- Content Negotiation: Smart language detection hierarchies
SEO and Multilingual Content
Properly handling internationalized content has significant SEO implications:
Best practices for multilingual SEO include:
- URL Structure: Consistent language indicators in URLs
- Hreflang Tags: Proper cross-linking between language versions
- Content Translation: Full translation rather than machine translation
- Language-Specific Metadata: Translated titles, descriptions, and keywords
- Structured Data: Localized schema.org markup
- Content Targeting: Culturally adapted content, not just translated
- Canonical Links: Clear indication of primary language versions
# views.py
from django.shortcuts import render
from django.utils.translation import get_language
def product_detail(request, product_id):
product = get_product(product_id)
current_language = get_language()
# Generate alternate URLs for all supported languages
languages = settings.LANGUAGES
alternate_urls = {}
for lang_code, lang_name in languages:
alternate_urls[lang_code] = request.build_absolute_uri(
f'/{lang_code}/product/{product_id}/'
)
return render(request, 'product_detail.html', {
'product': product,
'alternate_urls': alternate_urls,
})
# product_detail.html
{% for lang_code, url in alternate_urls.items %}
{% endfor %}
Testing and Quality Assurance
Internationalization requires specific testing approaches:
- Pseudo-Localization: Testing with automatically modified strings that simulate translation effects
- Expansion Testing: Some languages require much more space than English
- RTL Testing: Specific layout and flow testing
- Character Encoding: Ensuring proper handling of special characters
- Formatting Tests: Date, time, number, and currency format testing
- Translation Context: Ensuring translations work in application context
- Performance Testing: Loading multiple language packs
from django.test import TestCase
from django.utils import translation
class InternationalizationTests(TestCase):
def test_home_page_in_english(self):
with translation.override('en'):
response = self.client.get('/')
self.assertContains(response, 'Welcome to our store')
def test_home_page_in_french(self):
with translation.override('fr'):
response = self.client.get('/fr/')
self.assertContains(response, 'Bienvenue dans notre magasin')
def test_product_price_formatting(self):
product_id = 1
with translation.override('en'):
response = self.client.get(f'/en/product/{product_id}/')
self.assertContains(response, '$10.99')
with translation.override('fr'):
response = self.client.get(f'/fr/produit/{product_id}/')
self.assertContains(response, '10,99 €')
def test_date_formatting(self):
with translation.override('en'):
response = self.client.get('/en/blog/')
self.assertContains(response, 'April 11, 2025')
with translation.override('de'):
response = self.client.get('/de/blog/')
self.assertContains(response, '11. April 2025')
Current Best Practices and Future Trends
Current Best Practices
- Translation as Code: Treating translations as part of the codebase, with PRs and reviews
- Continuous Localization: Automated workflows integrated with CI/CD
- String Context: Providing screenshots and descriptions for translators
- Placeholders over Concatenation: Using variables instead of combining strings
- Unicode/ICU Message Format: Standardized format for complex translations
- Component-Based Translations: Scoping translations to components
- Runtime Language Switching: Without full page reloads
- CSS Logical Properties: For better RTL support
- Cultural Adaptation: Not just translation but cultural context
Emerging Trends and Future Directions
- AI Translation Integration: Smarter machine translation with context awareness
- Real-time Translation APIs: Dynamic content translation
- Voice Interface Localization: Multilingual voice interactions
- Personalized Language Experience: User-specific dialect and terminology preferences
- Cultural UX Adaptation: Beyond language to cultural preferences in UX
- Context-Aware Translation Workflows: AI understanding of application context
- Universal Intl API Support: Better browser support for internationalization
- Translation Memory in Browser: Client-side reuse of translations
Conclusion
Internationalization and localization have evolved from afterthoughts to critical components of modern web frameworks. What began as simple language file swapping has become a sophisticated ecosystem of tools and practices that enable truly global applications. Effective i18n/l10n is no longer just about translation—it encompasses cultural adaptation, formatting conventions, layout considerations, and comprehensive testing strategies.
As the web continues to connect people across linguistic and cultural boundaries, internationalization capabilities will remain an essential part of framework development. The most successful frameworks provide not just tools for translation but comprehensive solutions for creating inclusive, accessible, and culturally appropriate user experiences for everyone, regardless of language or location.