Evolution of Form Processing in Web Applications
Forms have been the backbone of web interactivity since the earliest days of the internet. From simple contact forms to complex multi-step wizards, the evolution of form processing reflects the broader development of web technologies and frameworks. This article explores how form handling has evolved from basic CGI scripts to sophisticated framework-integrated systems.
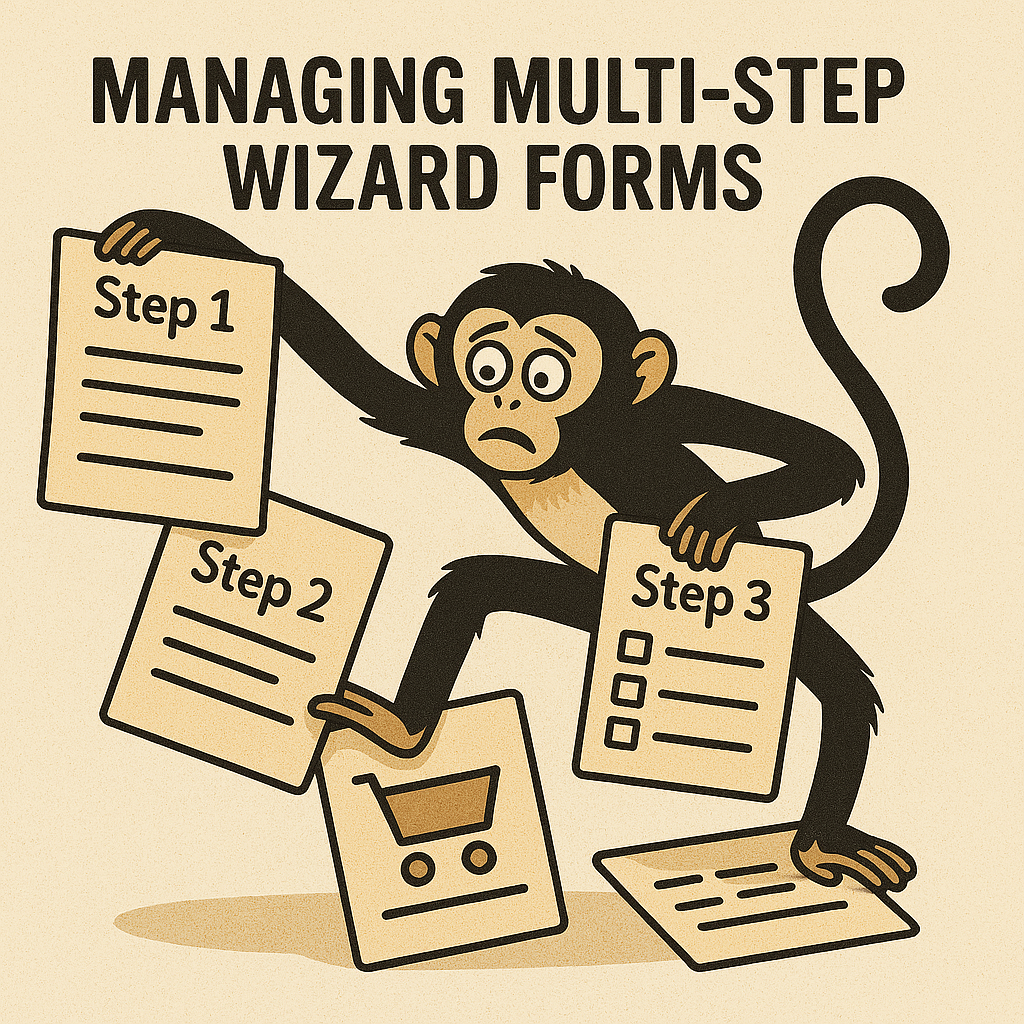
The evolution of form processing techniques across web development eras
The Early Days: CGI and Basic HTML Forms
In the early web (circa 1993-1997), nearly every interactive site was essentially a form-processing engine. Social networks, forums, and even basic e-commerce all relied on the same fundamental pattern:
- User fills out an HTML form
- Browser sends form data to the server via POST
- A CGI script (often written in Perl or C) processes the input
- Script writes results to a flat file or simple database
- Server generates a new HTML page with results or confirmation
#!/usr/bin/perl
use CGI;
# Create CGI object
my $cgi = new CGI;
# Get form values
my $name = $cgi->param('name');
my $email = $cgi->param('email');
my $message = $cgi->param('message');
# Validate inputs (very basic)
if (!$name || !$email || !$message) {
print $cgi->header;
print "Error: All fields are required. Please go back and try again.";
exit;
}
# Save to file
open(FH, ">>messages.txt") or die "Cannot open file: $!";
print FH "Name: $name\nEmail: $email\nMessage: $message\n\n";
close(FH);
# Output success page
print $cgi->header;
print <<HTML;
<html>
<head><title>Thank You</title></head>
<body>
<h1>Thank You!</h1>
<p>Your message has been received.</p>
<p><a href="index.html">Return to home page</a></p>
</body>
</html>
HTML
Challenges with this approach included:
- Limited validation capabilities
- Manual escaping to prevent security issues
- Repetitive code for similar forms
- No standardized approach to error handling
- Difficult to implement multi-page forms ("wizards")
Server-Side Frameworks: The PHP and ASP Era
As PHP (1995) and ASP (1996) gained popularity, form processing became more integrated with the page generation:
<?php
// Check if form was submitted
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// Collect form data
$name = $_POST['name'];
$email = $_POST['email'];
$message = $_POST['message'];
// Validate inputs
$errors = array();
if (empty($name)) {
$errors[] = "Name is required";
}
if (empty($email)) {
$errors[] = "Email is required";
} elseif (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
$errors[] = "Invalid email format";
}
if (empty($message)) {
$errors[] = "Message is required";
}
// Process form if no errors
if (empty($errors)) {
// Save to database
$conn = mysqli_connect("localhost", "user", "password", "feedback_db");
$sql = "INSERT INTO messages (name, email, message) VALUES (?, ?, ?)";
$stmt = mysqli_prepare($conn, $sql);
mysqli_stmt_bind_param($stmt, "sss", $name, $email, $message);
mysqli_stmt_execute($stmt);
mysqli_close($conn);
// Redirect to thank you page
header("Location: thank_you.php");
exit;
}
}
?>
<!-- HTML Form with error handling -->
<html>
<head><title>Contact Us</title></head>
<body>
<h1>Contact Us</h1>
<?php if (!empty($errors)): ?>
<div style="color: red;">
<ul>
<?php foreach ($errors as $error): ?>
<li><?php echo $error; ?></li>
<?php endforeach; ?>
</ul>
</div>
<?php endif; ?>
<form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>">
<div>
<label for="name">Name:</label>
<input type="text" id="name" name="name" value="<?php echo isset($name) ? $name : ''; ?>">
</div>
<div>
<label for="email">Email:</label>
<input type="email" id="email" name="email" value="<?php echo isset($email) ? $email : ''; ?>">
</div>
<div>
<label for="message">Message:</label>
<textarea id="message" name="message"><?php echo isset($message) ? $message : ''; ?></textarea>
</div>
<div>
<input type="submit" value="Submit">
</div>
</form>
</body>
</html>
Key improvements during this era:
- Form processing and display in the same file
- Better error handling with validation
- More sophisticated database integration
- Value persistence after validation failures
- Reusable include files for common form functions
However, the approach was still very manual and repetitive, with form processing logic tightly coupled with presentation.
The Rise of MVC Frameworks: Form Abstractions
With the rise of MVC frameworks like Ruby on Rails (2004), Django (2005), and ASP.NET MVC (2007), form processing became a first-class citizen in the development process:
# forms.py
from django import forms
class ContactForm(forms.Form):
name = forms.CharField(max_length=100, required=True)
email = forms.EmailField(required=True)
message = forms.CharField(widget=forms.Textarea, required=True)
def clean_email(self):
email = self.cleaned_data.get('email')
if email and not email.endswith('.com'):
raise forms.ValidationError("Only .com email addresses are accepted")
return email
# views.py
from django.shortcuts import render, redirect
from .forms import ContactForm
from .models import Message
def contact_view(request):
if request.method == 'POST':
form = ContactForm(request.POST)
if form.is_valid():
# Create and save a message object
Message.objects.create(
name=form.cleaned_data['name'],
email=form.cleaned_data['email'],
message=form.cleaned_data['message']
)
return redirect('thank_you')
else:
form = ContactForm()
return render(request, 'contact.html', {'form': form})
# template: contact.html
# Template code:
# <form method="post">
# {# csrf_token #}
# {# form.as_p #}
# <button type="submit">Submit</button>
# </form>
These frameworks introduced several paradigm shifts:
- Form Objects: Dedicated classes for form definition, validation, and rendering
- Automatic HTML Generation: Forms could render themselves as HTML
- Validation Rules: Declarative validation attached to fields
- Model Binding: Forms could be automatically created from data models
- CSRF Protection: Built-in security against cross-site request forgery
- Field Type System: Different field types with appropriate HTML widgets
The introduction of wizards and multi-step forms became significantly easier:
# Python code for a multi-step form wizard
from formtools.wizard.views import SessionWizardView
from django.shortcuts import redirect
from .forms import ContactDetailsForm, MessageForm, PreferencesForm
class ContactWizard(SessionWizardView):
form_list = [ContactDetailsForm, MessageForm, PreferencesForm]
template_name = 'contact_wizard.html'
def done(self, form_list, **kwargs):
# Process the completed forms
form_data = [form.cleaned_data for form in form_list]
# Create contact from all wizard steps
contact = Contact.objects.create(
name=form_data[0]['name'],
email=form_data[0]['email'],
message=form_data[1]['message'],
subscription=form_data[2]['subscribe']
)
return redirect('wizard_complete')
Modern JavaScript Frameworks: Client-Side Form Handling
With the emergence of React, Angular, and Vue.js, form processing shifted increasingly to the client-side:
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
import * as Yup from 'yup';
import axios from 'axios';
// Form validation schema
const ContactSchema = Yup.object().shape({
name: Yup.string()
.min(2, 'Too Short!')
.max(50, 'Too Long!')
.required('Required'),
email: Yup.string()
.email('Invalid email')
.required('Required'),
message: Yup.string()
.min(10, 'Message too short')
.required('Required'),
});
const ContactForm = () => {
return (
<div>
<h1>Contact Us</h1>
<Formik
initialValues={{ name: '', email: '', message: '' }}
validationSchema={ContactSchema}
onSubmit={(values, { setSubmitting, resetForm }) => {
// Submit to backend API
axios.post('/api/contact', values)
.then(response => {
alert('Thank you for your message!');
resetForm();
})
.catch(error => {
alert('There was an error submitting your form');
console.error(error);
})
.finally(() => {
setSubmitting(false);
});
}}
>
{({ isSubmitting }) => (
<Form>
<div>
<label htmlFor="name">Name</label>
<Field type="text" name="name" />
<ErrorMessage name="name" component="div" className="error" />
</div>
<div>
<label htmlFor="email">Email</label>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" className="error" />
</div>
<div>
<label htmlFor="message">Message</label>
<Field as="textarea" name="message" />
<ErrorMessage name="message" component="div" className="error" />
</div>
<button type="submit" disabled={isSubmitting}>
{isSubmitting ? 'Submitting...' : 'Submit'}
</button>
</Form>
)}
</Formik>
</div>
);
};
export default ContactForm;
The client-side revolution introduced:
- Real-time Validation: Immediate feedback as users type
- Declarative Form Libraries: Formik, React Hook Form, Angular Forms
- JSON APIs: Forms now submit to API endpoints instead of traditional form posts
- Controlled Components: Form state managed by JavaScript
- Enhanced UX: Dynamic form elements that appear/disappear based on user input
- Asynchronous Submission: No page reloads necessary
Form Processing Evolution Timeline
Era | Technologies | Key Form Processing Features |
---|---|---|
1993-1997 | CGI, Perl, C | Basic form processing, minimal validation, flat file storage |
1998-2004 | PHP, ASP, JSP | Integrated page generation, manual validation, database storage |
2005-2010 | Rails, Django, ASP.NET MVC | Form objects, model binding, declarative validation, CSRF protection |
2011-2015 | jQuery, Backbone, Angular | Client-side validation, AJAX submission, early SPA forms |
2016-Present | React, Vue, Angular | Controlled components, real-time validation, state management, form libraries |
Future | AI-Enhanced Frameworks | Smart form generation, predictive inputs, context-aware validation |
Data-to-Form Mapping: Reducing Boilerplate
One of the most significant evolutions has been the reduction of boilerplate code through automatic mapping between data models and forms:
# models.py
from django.db import models
class Contact(models.Model):
name = models.CharField(max_length=100)
email = models.EmailField()
phone = models.CharField(max_length=15, blank=True)
message = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return f"{self.name} ({self.email})"
# forms.py
from django import forms
from .models import Contact
class ContactModelForm(forms.ModelForm):
class Meta:
model = Contact
fields = ['name', 'email', 'phone', 'message']
widgets = {
'message': forms.Textarea(attrs={'rows': 5}),
}
def clean_phone(self):
phone = self.cleaned_data.get('phone')
# Custom validation logic
return phone
# views.py
def contact_view(request):
if request.method == 'POST':
form = ContactModelForm(request.POST)
if form.is_valid():
# Form automatically creates model instance
form.save()
return redirect('thank_you')
else:
form = ContactModelForm()
return render(request, 'contact.html', {'form': form})
This pattern has been replicated across frameworks:
- Django: ModelForms automatically create forms from models
- Laravel: Form requests with built-in validation
- Ruby on Rails: ActiveModel forms with automatic mapping
- Spring Boot: @ModelAttribute binding
- ASP.NET Core: Model binding with validation attributes
- React/GraphQL: Form generation from schema definitions
Modern Form UI Components and Design Systems
Today's form handling is increasingly characterized by sophisticated UI component libraries:
- Material UI: Google's design system with complex form controls
- Ant Design: Enterprise-grade UI system with extensive form capabilities
- Chakra UI: Accessible form components with modern styling
- TailwindCSS: Utility-first approach to form styling
- shadcn/ui: Composable, accessible components for forms
These systems offer:
- Consistent form styling and behavior
- Accessibility built-in (ARIA, keyboard navigation)
- Complex input types (date pickers, autocomplete, dropdowns)
- Mobile-friendly inputs
- Dark mode support
- Animations and visual feedback
Current Challenges and Future Directions
Despite decades of evolution, form processing still presents challenges:
- Complexity Management: Large forms with complex validation still require significant code
- Mobile Experience: Optimizing forms for small screens remains challenging
- Accessibility: Making forms usable for all remains an ongoing effort
- Performance: Complex forms can impact application performance
- Multi-step Processes: Managing state across multi-page wizards
- Server-Client Validation Consistency: Keeping rules synchronized
Future directions include:
- AI-Enhanced Forms: Smart suggestions, auto-fill, and context-aware validation
- Voice and Natural Language Inputs: Beyond traditional keyboard entry
- Cross-Device Experiences: Start on mobile, continue on desktop
- No-Code Form Builders: More sophisticated drag-and-drop form creation
- Form Schema Standards: Universal specifications for form definitions
- Biometric and Secure Inputs: Fingerprint, face ID integration
Conclusion
The evolution of form processing in web applications reflects the broader journey of web development itself—from simple CGI scripts to sophisticated framework ecosystems. Forms remain the primary interface between users and web applications, making their implementation a crucial aspect of web development.
Modern frameworks have dramatically reduced the effort required to create, validate, and process forms, allowing developers to focus on user experience rather than technical implementation. As web technologies continue to advance, we can expect form processing to evolve with increasing intelligence, adaptability, and sophistication.