Evolution of Deployment & DevOps Integration
Web application deployment has transformed dramatically over the past three decades, evolving from manual file transfers and shell scripts to sophisticated containerized environments and declarative infrastructure. This article explores the historical progression of deployment practices, configuration management, and infrastructure automation, examining how each phase introduced new capabilities while creating new challenges.
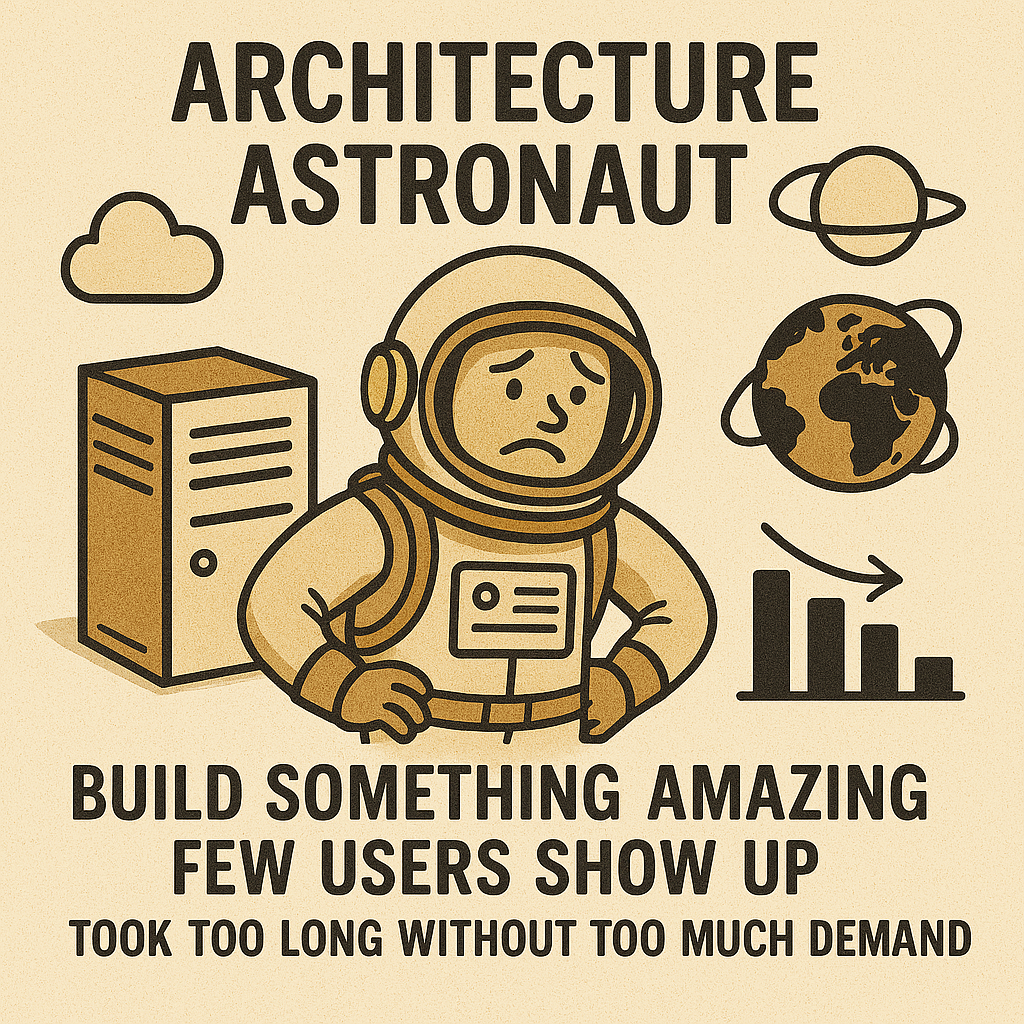
The evolution of deployment practices from manual operations to modern automated approaches
The Early Days: Manual Processes & Shell Scripts (1990s-early 2000s)
In the early days of web development, deployment was largely a manual process with minimal automation:
Common Practices
- Manual FTP file transfers
- Basic shell scripts for repetitive tasks
- Direct server modifications via SSH/Telnet
- Handwritten deployment documentation
- Manual database schema changes
- Physical backups onto tape drives
Key Challenges
- Error-prone manual processes
- Inconsistent environments
- Limited rollback capabilities
- Frequent configuration drift
- Long recovery times after failures
- Knowledge siloed in individual operators
#!/bin/bash
# Simple deployment script circa 2001
# Run as: ./deploy.sh production
ENV=$1
TIMESTAMP=$(date +%Y%m%d_%H%M%S)
BACKUP_DIR="/backup/${ENV}_${TIMESTAMP}"
echo "Starting deployment to $ENV environment at $(date)"
echo "Creating backup directory $BACKUP_DIR"
mkdir -p $BACKUP_DIR
# Back up current application
echo "Backing up current application..."
tar -czf $BACKUP_DIR/app_backup.tar.gz /var/www/html/
# Back up database
echo "Backing up database..."
mysqldump -u root -p$DB_PASSWORD myapp_db > $BACKUP_DIR/db_backup.sql
# Stop web server
echo "Stopping Apache..."
/etc/init.d/apache stop
# Deploy new code
echo "Copying new files..."
cp -R /tmp/release/* /var/www/html/
# Run database migrations
echo "Running database updates..."
mysql -u root -p$DB_PASSWORD myapp_db < /var/www/html/db/migrations.sql
# Fix permissions
echo "Setting file permissions..."
chown -R www-data:www-data /var/www/html/
find /var/www/html/ -type d -exec chmod 755 {} \;
find /var/www/html/ -type f -exec chmod 644 {} \;
# Start web server
echo "Starting Apache..."
/etc/init.d/apache start
echo "Deployment completed at $(date)"
This era was characterized by:
- Disaster Recovery Documents: Detailed step-by-step recovery procedures, often stored in binders or text files
- Backup Rotation Schemes: Complex systems for tape rotation and offsite storage
- Institutional Knowledge: Critical system information lived primarily in experienced operators' minds
- Environment Uniqueness: Production environments were often one-of-a-kind, manually constructed systems
The Rise of Configuration Management (Mid-2000s to Early 2010s)
The pain points of manual deployments led to the rise of configuration management tools designed to make server provisioning and application deployment more consistent and automated:
Tool | Released | Key Features | Limitations | Legacy Today |
---|---|---|---|---|
CFEngine | 1993 (v3 in 2008) | Lightweight agent, promise theory, C-based | Complex syntax, steep learning curve | Still used in legacy enterprise systems |
Puppet | 2005 | Declarative DSL, resource modeling, Ruby-based | Master-agent architecture complexity, performance | Declining usage but remains in enterprises |
Chef | 2009 | Procedural Ruby DSL, "recipes", dev-friendly | Complexity for simple tasks, Ruby dependency | Continued but declining usage |
Ansible | 2012 | Agentless, YAML-based, SSH-driven | Performance at scale, execution flow complexity | Still widely used, especially for simpler environments |
SaltStack | 2011 | Event-driven, high-speed, Python-based | Complex setup, documentation gaps | Maintained usage in specific sectors |
The Puppet Phenomenon and Decline
Puppet represented a significant paradigm shift in infrastructure management, introducing:
- Infrastructure as Code: Defining server configuration in version-controlled files
- Declarative Model: Specifying desired state, not procedural steps
- Idempotent Operations: Commands that can be run repeatedly with the same result
- Configuration Catalogs: Centralized management of environment configuration
However, Puppet's decline can be attributed to several factors:
- Complex client-server architecture requiring significant maintenance
- Rise of cloud platforms with native provisioning capabilities
- Emergence of container technologies that packaged configuration with applications
- Shift toward simpler, agentless tools like Ansible
- Performance issues when managing thousands of nodes
# Example Puppet manifest for a web server circa 2010
node 'webserver.example.com' {
# Ensure Apache is installed and running
package { 'apache2':
ensure => installed,
}
service { 'apache2':
ensure => running,
enable => true,
require => Package['apache2'],
}
# Configure virtual host
file { '/etc/apache2/sites-available/myapp.conf':
ensure => file,
content => template('myapp/vhost.erb'),
require => Package['apache2'],
notify => Service['apache2'],
}
# Enable the site
exec { 'enable-myapp-site':
command => '/usr/sbin/a2ensite myapp.conf',
creates => '/etc/apache2/sites-enabled/myapp.conf',
require => File['/etc/apache2/sites-available/myapp.conf'],
notify => Service['apache2'],
}
# Deploy application code
file { '/var/www/myapp':
ensure => directory,
owner => 'www-data',
group => 'www-data',
mode => '0755',
recurse => true,
source => 'puppet:///modules/myapp/code',
require => Package['apache2'],
}
# Install database client
package { 'mysql-client':
ensure => installed,
}
# Install PHP and extensions
$php_packages = [
'php5',
'php5-mysql',
'php5-gd',
'libapache2-mod-php5',
]
package { $php_packages:
ensure => installed,
require => Package['apache2'],
notify => Service['apache2'],
}
}
Ansible: The Blueprint for Portable Configuration Management
Ansible emerged as a response to the complexity of earlier tools, with several key innovations:
- Agentless architecture using SSH for communication
- YAML-based playbooks that were simpler to read and write
- Push-based execution model (vs. pull-based in Puppet/Chef)
- Minimal dependencies on managed systems
- Flatter learning curve for operators
- Easy integration with existing shell scripts and commands
# Example Ansible playbook circa 2013
---
- name: Configure web servers
hosts: webservers
become: yes
tasks:
- name: Install Apache
apt:
name: apache2
state: present
update_cache: yes
- name: Ensure Apache is running
service:
name: apache2
state: started
enabled: yes
- name: Deploy virtual host configuration
template:
src: templates/vhost.conf.j2
dest: /etc/apache2/sites-available/myapp.conf
notify: Reload Apache
- name: Enable site
command: a2ensite myapp.conf
args:
creates: /etc/apache2/sites-enabled/myapp.conf
notify: Reload Apache
- name: Create application directory
file:
path: /var/www/myapp
state: directory
owner: www-data
group: www-data
mode: '0755'
- name: Deploy application code
copy:
src: files/app/
dest: /var/www/myapp
owner: www-data
group: www-data
notify: Reload Apache
- name: Install PHP and extensions
apt:
name: "{{ item }}"
state: present
with_items:
- php5
- php5-mysql
- php5-gd
- libapache2-mod-php5
notify: Restart Apache
handlers:
- name: Reload Apache
service:
name: apache2
state: reloaded
- name: Restart Apache
service:
name: apache2
state: restarted
The Virtualization Era: From Physical to Virtual (Mid-2000s to Mid-2010s)
The widespread adoption of virtualization technologies transformed deployment practices by introducing flexibility and resource efficiency:
Key Developments
- Hardware Virtualization: VMware ESX/ESXi, Xen, KVM
- Virtual Machine Templates: Golden images for consistent deployment
- VM Snapshots: Point-in-time system state capture
- Resource Overcommitment: Running more VMs than physical capacity
- Live Migration: Moving running VMs between hosts
Deployment Impact
- Faster provisioning of new environments
- Clearer separation between applications
- Better disaster recovery options
- Decreased hardware costs through consolidation
- Environment cloning for testing deployments
- Rise of horizontally scaled application architectures
The Golden Image Approach
Many organizations adopted a "golden image" deployment strategy:
- Create a base VM with the OS and common software
- Configure application-specific requirements
- Create a snapshot or template
- Deploy multiple instances from the template
- Apply environment-specific configuration on first boot
#!/bin/bash
# Example first-boot configuration script for a VM circa 2010
# This would typically be run by cloud-init or similar
# Read environment from metadata service or configuration file
ENVIRONMENT=$(curl -s http://169.254.169.254/latest/meta-data/environment)
APP_VERSION=$(curl -s http://169.254.169.254/latest/meta-data/app-version)
DB_HOST=$(curl -s http://169.254.169.254/latest/meta-data/db-host)
# Configure hostname based on environment
HOSTNAME="web-${ENVIRONMENT}-$(hostname | cut -d'-' -f2)"
hostname $HOSTNAME
echo $HOSTNAME > /etc/hostname
echo "127.0.0.1 $HOSTNAME localhost" > /etc/hosts
# Update application configuration
CONFIG_FILE="/var/www/myapp/config.php"
sed -i "s/DB_HOST = '.*'/DB_HOST = '$DB_HOST'/g" $CONFIG_FILE
sed -i "s/APP_ENV = '.*'/APP_ENV = '$ENVIRONMENT'/g" $CONFIG_FILE
# Pull latest application code if needed
if [ "$ENVIRONMENT" != "production" ]; then
cd /var/www/myapp
git pull origin $APP_VERSION
chown -R www-data:www-data /var/www/myapp
fi
# Register with load balancer
echo "Registering with load balancer..."
curl -X POST "http://lb-manager.internal/register?host=$(hostname -I | awk '{print $1}')"
# Start application services
systemctl start myapp-worker
systemctl start myapp-scheduler
echo "First boot configuration completed at $(date)" >> /var/log/firstboot.log
Virtualization Challenges
Despite its benefits, virtualization introduced new challenges:
- VM Sprawl: Uncontrolled proliferation of virtual machines
- Golden Image Maintenance: Keeping base images updated and patched
- Configuration Drift: VMs diverging from their original state over time
- Resource Contention: Performance issues from overcommitment
- Complex Licensing: Software licensing complications in virtual environments
The Cloud Era: Infrastructure as a Service (2006 onwards)
Cloud platforms introduced API-driven infrastructure provisioning that fundamentally changed deployment practices:
Key Cloud Innovations
- API-driven Infrastructure: Programmatic resource creation
- Auto Scaling: Dynamic adjustment of capacity
- Elastic Load Balancing: Traffic distribution across instances
- Pay-as-you-go Pricing: Usage-based billing
- Regions & Availability Zones: Geographic distribution
- Managed Services: Databases, caching, queuing as services
Deployment Impact
- Self-service provisioning for development teams
- Faster time-to-market for applications
- Infrastructure that scales with demand
- Reduced upfront capital expenditure
- Global deployment capabilities
- Standardized infrastructure APIs
The CloudFormation/Terraform Revolution
Cloud providers introduced Infrastructure as Code (IaC) tools that allowed complete environments to be defined in declarative templates:
Tool | Provider | Approach | Strengths | Limitations |
---|---|---|---|---|
CloudFormation | AWS | JSON/YAML templates, stack-based deployment | Deep AWS integration, managed state | AWS-specific, complex templates |
Azure Resource Manager | Azure | JSON templates, resource groups | Azure ecosystem integration | Azure-specific, verbose syntax |
Google Deployment Manager | GCP | YAML templates with Python/Jinja2 | GCP integration, flexible templating | GCP-specific, less mature ecosystem |
Terraform | Multi-cloud | HCL syntax, provider plugins | Cross-cloud, provider ecosystem | State management complexity, abstraction limitations |
Pulumi | Multi-cloud | General-purpose languages (Python, TS, etc.) | Full programming languages, testability | Learning curve, newer platform |
AWSTemplateFormatVersion: '2010-09-09'
Description: 'Web application stack with auto scaling'
Parameters:
EnvironmentName:
Type: String
Default: Development
AllowedValues: [Development, Staging, Production]
Description: Environment name
InstanceType:
Type: String
Default: t3.small
AllowedValues: [t3.small, t3.medium, t3.large]
Description: EC2 instance type
Resources:
VPC:
Type: AWS::EC2::VPC
Properties:
CidrBlock: 10.0.0.0/16
EnableDnsSupport: true
EnableDnsHostnames: true
Tags:
- Key: Name
Value: !Sub ${EnvironmentName}-VPC
PublicSubnet1:
Type: AWS::EC2::Subnet
Properties:
VpcId: !Ref VPC
AvailabilityZone: !Select [0, !GetAZs '']
CidrBlock: 10.0.1.0/24
MapPublicIpOnLaunch: true
Tags:
- Key: Name
Value: !Sub ${EnvironmentName}-PublicSubnet1
PublicSubnet2:
Type: AWS::EC2::Subnet
Properties:
VpcId: !Ref VPC
AvailabilityZone: !Select [1, !GetAZs '']
CidrBlock: 10.0.2.0/24
MapPublicIpOnLaunch: true
Tags:
- Key: Name
Value: !Sub ${EnvironmentName}-PublicSubnet2
WebServerSecurityGroup:
Type: AWS::EC2::SecurityGroup
Properties:
GroupDescription: Allow HTTP and SSH access
VpcId: !Ref VPC
SecurityGroupIngress:
- IpProtocol: tcp
FromPort: 80
ToPort: 80
CidrIp: 0.0.0.0/0
- IpProtocol: tcp
FromPort: 443
ToPort: 443
CidrIp: 0.0.0.0/0
- IpProtocol: tcp
FromPort: 22
ToPort: 22
CidrIp: 0.0.0.0/0
WebServerLaunchConfig:
Type: AWS::AutoScaling::LaunchConfiguration
Properties:
ImageId: ami-0abcdef1234567890 # Amazon Linux 2 AMI
InstanceType: !Ref InstanceType
SecurityGroups:
- !Ref WebServerSecurityGroup
UserData:
Fn::Base64: !Sub |
#!/bin/bash -xe
yum update -y
yum install -y httpd
echo "Hello from ${EnvironmentName}
" > /var/www/html/index.html
systemctl start httpd
systemctl enable httpd
WebServerGroup:
Type: AWS::AutoScaling::AutoScalingGroup
Properties:
VPCZoneIdentifier:
- !Ref PublicSubnet1
- !Ref PublicSubnet2
LaunchConfigurationName: !Ref WebServerLaunchConfig
MinSize: 2
MaxSize: 5
DesiredCapacity: 2
Tags:
- Key: Name
Value: !Sub ${EnvironmentName}-WebServer
PropagateAtLaunch: true
Outputs:
VPC:
Description: VPC ID
Value: !Ref VPC
PublicSubnets:
Description: Public subnet IDs
Value: !Join [", ", [!Ref PublicSubnet1, !Ref PublicSubnet2]]
provider "aws" {
region = "us-west-2"
}
variable "environment" {
description = "Deployment environment"
default = "development"
}
resource "aws_vpc" "main" {
cidr_block = "10.0.0.0/16"
enable_dns_support = true
enable_dns_hostnames = true
tags = {
Name = "${var.environment}-vpc"
Environment = var.environment
}
}
resource "aws_subnet" "public_a" {
vpc_id = aws_vpc.main.id
cidr_block = "10.0.1.0/24"
availability_zone = "us-west-2a"
map_public_ip_on_launch = true
tags = {
Name = "${var.environment}-public-a"
Environment = var.environment
}
}
resource "aws_subnet" "public_b" {
vpc_id = aws_vpc.main.id
cidr_block = "10.0.2.0/24"
availability_zone = "us-west-2b"
map_public_ip_on_launch = true
tags = {
Name = "${var.environment}-public-b"
Environment = var.environment
}
}
resource "aws_security_group" "web" {
name = "${var.environment}-web-sg"
description = "Security group for web servers"
vpc_id = aws_vpc.main.id
ingress {
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
ingress {
from_port = 443
to_port = 443
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
resource "aws_launch_configuration" "web" {
name_prefix = "${var.environment}-web-"
image_id = "ami-0abcdef1234567890"
instance_type = "t3.small"
security_groups = [aws_security_group.web.id]
user_data = <<-EOF
#!/bin/bash
yum update -y
yum install -y httpd
echo "Hello from ${var.environment}
" > /var/www/html/index.html
systemctl start httpd
systemctl enable httpd
EOF
lifecycle {
create_before_destroy = true
}
}
resource "aws_autoscaling_group" "web" {
name = "${var.environment}-web-asg"
launch_configuration = aws_launch_configuration.web.name
min_size = 2
max_size = 5
desired_capacity = 2
vpc_zone_identifier = [aws_subnet.public_a.id, aws_subnet.public_b.id]
tag {
key = "Name"
value = "${var.environment}-web"
propagate_at_launch = true
}
tag {
key = "Environment"
value = var.environment
propagate_at_launch = true
}
lifecycle {
create_before_destroy = true
}
}
Vendor Lock-in Challenges
While cloud provider-specific tools like CloudFormation offered deep integration, they introduced significant vendor lock-in concerns:
- Non-portable infrastructure definitions
- Reliance on provider-specific features
- Complex migration paths between cloud providers
- Skills specialization around specific platforms
- Dependency on provider's implementation timelines for new features
These concerns led to the rise of cross-cloud tools like Terraform and the Cloud Development Kit (CDK) approaches that offered more flexibility.
The Container Revolution: Docker & Beyond (2013 onwards)
Containers transformed application packaging and deployment by providing a consistent environment from development through production:
Container Innovations
- Consistent Runtime Environment: Same container from dev to prod
- Immutable Infrastructure: Replace rather than modify
- Lightweight Isolation: Efficient resource usage
- Layered File System: Efficient storage and distribution
- Application-centric Packaging: Application and dependencies together
- Fast Startup: Seconds vs. minutes for VMs
Deployment Impact
- "Works on my machine" problems largely eliminated
- Microservices architectures became practical
- Simplified continuous deployment pipelines
- Improved resource utilization
- Faster scaling operations
- Better local development environments
# Example Dockerfile for a web application
FROM node:14-alpine AS build
WORKDIR /app
# Copy package.json and install dependencies
COPY package*.json ./
RUN npm ci
# Copy application code
COPY . .
# Build the application
RUN npm run build
# Production image
FROM nginx:alpine
# Copy built assets from build stage
COPY --from=build /app/build /usr/share/nginx/html
# Copy custom nginx config
COPY nginx.conf /etc/nginx/conf.d/default.conf
# Expose port
EXPOSE 80
# Health check
HEALTHCHECK --interval=30s --timeout=3s \
CMD wget -q --spider http://localhost/ || exit 1
# Start nginx
CMD ["nginx", "-g", "daemon off;"]
Container Orchestration Evolution
Individual containers solved application packaging, but orchestration systems were needed to manage containers at scale:
Platform | Key Features | Primary Use Cases | Adoption Status |
---|---|---|---|
Docker Compose | Simple multi-container definition, development-focused | Local development, simple deployments | Widely used for development |
Docker Swarm | Cluster management, service scaling, overlay networking | Small to medium deployments | Declining, largely replaced by Kubernetes |
Kubernetes | Advanced orchestration, self-healing, extensive API | Complex, large-scale deployments | Industry standard for container orchestration |
Amazon ECS | AWS-integrated container service, task definitions | AWS-based container workloads | Popular for AWS-centric organizations |
Nomad | Lightweight, multi-workload orchestrator | Mixed container/non-container environments | Niche adoption, growing in specific sectors |
# Example Kubernetes deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: web-application
labels:
app: web
spec:
replicas: 3
selector:
matchLabels:
app: web
strategy:
rollingUpdate:
maxSurge: 1
maxUnavailable: 0
type: RollingUpdate
template:
metadata:
labels:
app: web
spec:
containers:
- name: web
image: mycompany/web-app:1.0.0
ports:
- containerPort: 80
resources:
limits:
cpu: "0.5"
memory: "512Mi"
requests:
cpu: "0.2"
memory: "256Mi"
livenessProbe:
httpGet:
path: /health
port: 80
initialDelaySeconds: 30
periodSeconds: 10
readinessProbe:
httpGet:
path: /ready
port: 80
initialDelaySeconds: 5
periodSeconds: 5
env:
- name: NODE_ENV
value: "production"
- name: DATABASE_URL
valueFrom:
secretKeyRef:
name: app-secrets
key: database-url
imagePullSecrets:
- name: registry-credentials
---
apiVersion: v1
kind: Service
metadata:
name: web-service
spec:
selector:
app: web
ports:
- port: 80
targetPort: 80
type: LoadBalancer
GitOps: The Git-Centric Deployment Model
Containers and Kubernetes enabled a new deployment paradigm called GitOps, characterized by:
- Git as Single Source of Truth: Infrastructure and app config in git
- Declarative Configurations: Desired state, not procedural steps
- Pull-Based Deployment: Agents reconcile actual vs. desired state
- Continuous Reconciliation: Automatic drift correction
- Full Audit Trail: Git history provides deployment tracking
Tools like Flux and ArgoCD emerged to implement the GitOps workflow, providing automated synchronization between Git repositories and Kubernetes clusters.
The Serverless Era: Functions and Managed Services (2014 onwards)
Serverless computing further abstracted infrastructure management, shifting focus entirely to application code:
Serverless Characteristics
- No Server Management: Infrastructure fully abstracted
- Pay-per-execution: No costs when idle
- Auto-scaling: Automatic capacity adjustment
- Event-driven: Functions triggered by events
- Stateless Execution: No persistent server context
- Managed Service Integration: Built-in connections to cloud services
Deployment Impact
- Focus on business logic rather than infrastructure
- Rapid development and deployment cycles
- Simplified operational management
- Cost optimization for variable workloads
- Built-in high availability
- Reduced operational overhead
# serverless.yml
service: user-api
provider:
name: aws
runtime: nodejs14.x
stage: ${opt:stage, 'dev'}
region: us-east-1
environment:
TABLE_NAME: ${self:service}-${self:provider.stage}-users
iamRoleStatements:
- Effect: Allow
Action:
- dynamodb:PutItem
- dynamodb:GetItem
- dynamodb:UpdateItem
- dynamodb:DeleteItem
- dynamodb:Scan
Resource: !GetAtt UsersTable.Arn
functions:
createUser:
handler: src/handlers/createUser.handler
events:
- http:
path: /users
method: post
cors: true
getUser:
handler: src/handlers/getUser.handler
events:
- http:
path: /users/{id}
method: get
cors: true
listUsers:
handler: src/handlers/listUsers.handler
events:
- http:
path: /users
method: get
cors: true
resources:
Resources:
UsersTable:
Type: AWS::DynamoDB::Table
Properties:
TableName: ${self:provider.environment.TABLE_NAME}
BillingMode: PAY_PER_REQUEST
AttributeDefinitions:
- AttributeName: id
AttributeType: S
KeySchema:
- AttributeName: id
KeyType: HASH
// src/handlers/createUser.js
const AWS = require('aws-sdk');
const { v4: uuidv4 } = require('uuid');
const dynamoDb = new AWS.DynamoDB.DocumentClient();
const tableName = process.env.TABLE_NAME;
exports.handler = async (event) => {
try {
const requestBody = JSON.parse(event.body);
const { name, email } = requestBody;
if (!name || !email) {
return {
statusCode: 400,
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ error: 'Name and email are required' })
};
}
const userId = uuidv4();
const timestamp = new Date().getTime();
const user = {
id: userId,
name,
email,
createdAt: timestamp,
updatedAt: timestamp
};
await dynamoDb.put({
TableName: tableName,
Item: user
}).promise();
return {
statusCode: 201,
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(user)
};
} catch (error) {
console.error('Error creating user:', error);
return {
statusCode: 500,
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ error: 'Could not create the user' })
};
}
};
Serverless Framework and CDK Approaches
To manage the complexity of serverless deployments, higher-level frameworks emerged:
- Serverless Framework: YAML-based definitions for multi-provider support
- AWS CDK: Infrastructure as actual code in TypeScript, Python, etc.
- SAM (Serverless Application Model): AWS-specific serverless template format
- Architect: Pragmatic JS/JSON-based serverless definition
import * as cdk from 'aws-cdk-lib';
import { Construct } from 'constructs';
import * as lambda from 'aws-cdk-lib/aws-lambda';
import * as apigateway from 'aws-cdk-lib/aws-apigateway';
import * as dynamodb from 'aws-cdk-lib/aws-dynamodb';
export class UserApiStack extends cdk.Stack {
constructor(scope: Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
// DynamoDB table
const userTable = new dynamodb.Table(this, 'Users', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
billingMode: dynamodb.BillingMode.PAY_PER_REQUEST,
removalPolicy: cdk.RemovalPolicy.DESTROY, // NOT for production
});
// Lambda functions
const createUserFunction = new lambda.Function(this, 'CreateUserFunction', {
runtime: lambda.Runtime.NODEJS_14_X,
handler: 'createUser.handler',
code: lambda.Code.fromAsset('src/handlers'),
environment: {
TABLE_NAME: userTable.tableName,
},
});
const getUserFunction = new lambda.Function(this, 'GetUserFunction', {
runtime: lambda.Runtime.NODEJS_14_X,
handler: 'getUser.handler',
code: lambda.Code.fromAsset('src/handlers'),
environment: {
TABLE_NAME: userTable.tableName,
},
});
const listUsersFunction = new lambda.Function(this, 'ListUsersFunction', {
runtime: lambda.Runtime.NODEJS_14_X,
handler: 'listUsers.handler',
code: lambda.Code.fromAsset('src/handlers'),
environment: {
TABLE_NAME: userTable.tableName,
},
});
// Grant permissions
userTable.grantReadWriteData(createUserFunction);
userTable.grantReadData(getUserFunction);
userTable.grantReadData(listUsersFunction);
// API Gateway
const api = new apigateway.RestApi(this, 'UserApi', {
restApiName: 'User Service',
description: 'API for managing users',
defaultCorsPreflightOptions: {
allowOrigins: apigateway.Cors.ALL_ORIGINS,
allowMethods: apigateway.Cors.ALL_METHODS,
},
});
const users = api.root.addResource('users');
users.addMethod('POST', new apigateway.LambdaIntegration(createUserFunction));
users.addMethod('GET', new apigateway.LambdaIntegration(listUsersFunction));
const user = users.addResource('{id}');
user.addMethod('GET', new apigateway.LambdaIntegration(getUserFunction));
// Outputs
new cdk.CfnOutput(this, 'ApiUrl', {
value: api.url,
description: 'URL of the API Gateway',
});
}
}
Current State & Future Trends
Modern deployment and DevOps integration has evolved into a sophisticated ecosystem that continues to develop rapidly:
The Contemporary Deployment Landscape
Current Best Practices
- GitOps Workflows: Git-driven deployment automation
- Infrastructure as Code: Declarative environment definitions
- CI/CD Pipelines: Automated build and deploy workflows
- Immutable Infrastructure: Replace rather than modify
- Observability Stacks: Integrated monitoring, logging, tracing
- Security Automation: Vulnerability scanning, compliance checks
- Multi-environment Promotion: Controlled progression through environments
Emerging Trends
- FinOps Integration: Cost management in deployment tooling
- AI-assisted Operations: Intelligent monitoring and remediation
- Platform Engineering: Internal developer platforms
- Policy as Code: Automated governance and compliance
- Low-code Deployment: Simplified deployment interfaces
- Edge Deployment: Distributing workloads closer to users
- Sustainability Metrics: Carbon impact of deployments
The Platform Engineering Evolution
The most significant current trend is the rise of platform engineering, which provides abstracted deployment capabilities to development teams:
- Internal Developer Platforms: Curated tools and workflows for developers
- Self-service Infrastructure: Abstracted access to resources via portals/APIs
- Golden Paths: Standardized deployment patterns for common use cases
- Service Catalogs: Pre-approved, easily consumable infrastructure components
- Backstage and Similar Tools: Developer portals for service management
This approach combines the flexibility of modern cloud-native deployments with the governance needs of enterprise organizations, creating a balance between developer autonomy and operational control.
Reflections on the Evolution
The deployment and DevOps landscape has evolved through several key paradigm shifts:
- From Manual to Automated: Replacing error-prone human tasks with reliable automation
- From Imperative to Declarative: Defining desired states rather than steps to achieve them
- From Pets to Cattle: Treating infrastructure as replaceable rather than unique
- From Monolithic to Microservices: Breaking down deployments into smaller, independent units
- From Long-lived to Ephemeral: Shorter lifespans for infrastructure components
- From Specialized to Generalized Skills: Broader responsibility for the full application lifecycle
Each phase has brought new capabilities but also introduced new challenges, requiring organizations to continuously adapt their deployment practices.
Conclusion
The evolution of deployment and DevOps integration reflects the broader technological shifts in computing over the past three decades. From manual file transfers and shell scripts to declarative infrastructure and GitOps workflows, each advancement has aimed to make deployments more reliable, efficient, and scalable.
Today's deployment landscape offers unprecedented flexibility and power, but also requires navigating a complex ecosystem of tools and approaches. Organizations must continually balance the adoption of new technologies against the pragmatic needs of their development teams and application portfolios.
As we look to the future, the integration between development and operations will likely become even more seamless, with increased abstraction hiding infrastructure complexity from developers while maintaining the governance and reliability requirements of modern applications.