Evolution of API Support in Web Development
APIs (Application Programming Interfaces) have become the backbone of modern web development, enabling everything from third-party integrations to microservice architectures. Yet the API landscape has evolved dramatically over the past few decades, with changing paradigms, standards, and practices. This article traces the historical evolution of API support in web development, exploring how we moved from simple CGI scripts to sophisticated GraphQL implementations, while highlighting the ongoing challenges of a fragmented ecosystem with diverse authentication methods, data formats, and billing models.
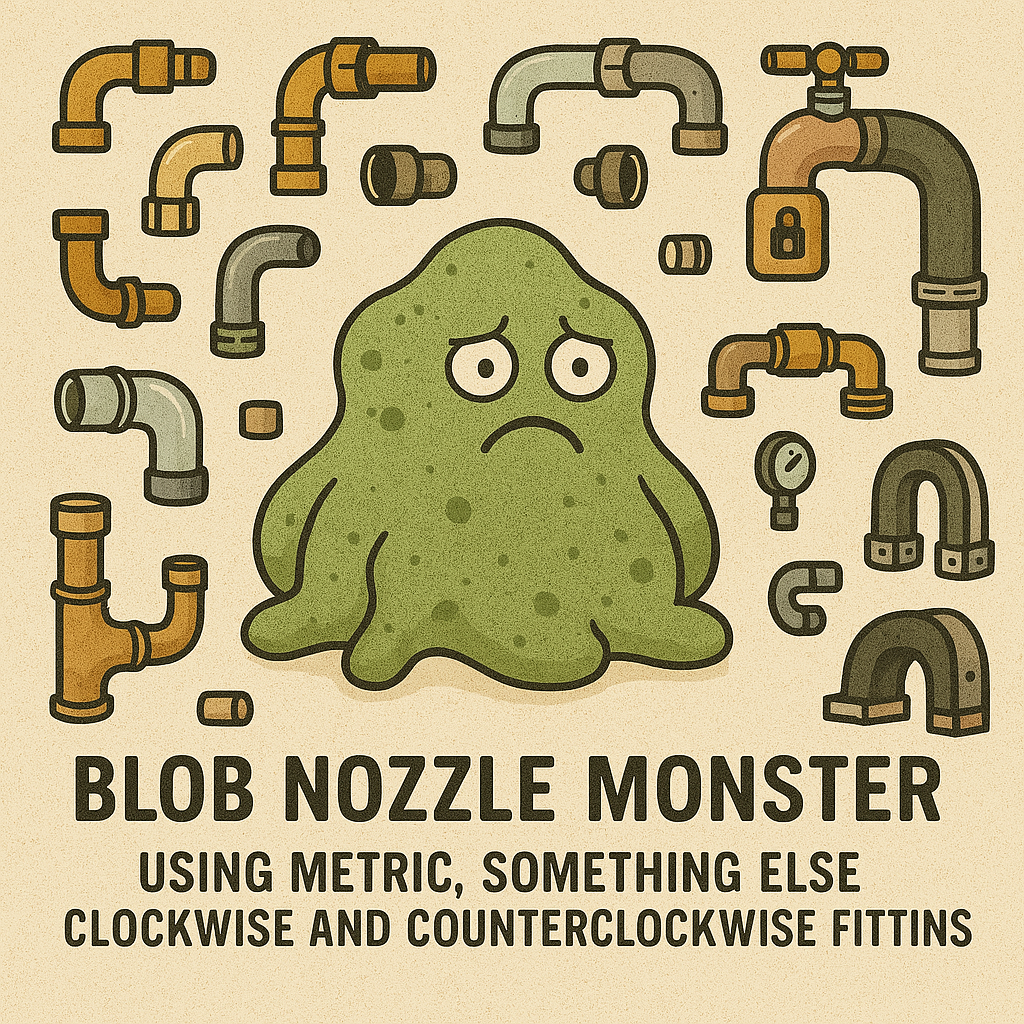
The evolution of API architectures in web development
The Early Days: RPC and CGI (1990s)
The earliest web APIs were primitive by today's standards, largely based on Remote Procedure Call (RPC) patterns and Common Gateway Interface (CGI) scripts:
Key Characteristics
- CGI Scripts: Perl and other scripts outputting HTML or simple data
- XML-RPC: Remote procedure calls using XML formatting
- Simple HTTP Calls: Basic GET/POST requests with minimal structure
- Proprietary Formats: Non-standardized data exchange formats
- Minimal Authentication: Basic auth or simple API keys
Common Challenges
- Limited serialization options
- Tight coupling between client and server
- Poor error handling
- Manual documentation
- Limited discoverability
- No versioning standards
<!-- XML-RPC Request -->
POST /RPC2 HTTP/1.0
User-Agent: XML-RPC Client
Host: api.example.com
Content-Type: text/xml
Content-Length: 181
<?xml version="1.0"?>
<methodCall>
<methodName>examples.getUser</methodName>
<params>
<param>
<value><int>123</int></value>
</param>
</params>
</methodCall>
<!-- XML-RPC Response -->
HTTP/1.1 200 OK
Server: Apache
Content-Type: text/xml
Content-Length: 358
<?xml version="1.0"?>
<methodResponse>
<params>
<param>
<value>
<struct>
<member>
<name>userId</name>
<value><int>123</int></value>
</member>
<member>
<name>username</name>
<value><string>johndoe</string></value>
</member>
<member>
<name>email</name>
<value><string>john@example.com</string></value>
</member>
</struct>
</value>
</param>
</params>
</methodResponse>
#!/usr/bin/perl
# Simple CGI API script (circa 1998)
use CGI;
use DBI;
use JSON;
print "Content-type: text/plain\n\n";
my $cgi = new CGI;
my $action = $cgi->param('action') || 'list';
my $id = $cgi->param('id') || '';
my $api_key = $cgi->param('api_key') || '';
# Extremely simple API key check
if ($api_key ne 'secret_key_12345') {
print "Invalid API Key";
exit;
}
# Connect to database
my $dbh = DBI->connect("DBI:mysql:database=products;host=localhost",
"user", "password", {'RaiseError' => 1});
if ($action eq 'list') {
my $sth = $dbh->prepare("SELECT id, name, price FROM products LIMIT 10");
$sth->execute();
my @products;
while (my $row = $sth->fetchrow_hashref) {
push @products, $row;
}
# Manual JSON creation - JSON module wasn't common yet
print "{\n";
print " \"products\": [\n";
my $first = 1;
foreach my $product (@products) {
print ",\n" unless $first;
$first = 0;
print " {\n";
print " \"id\": " . $product->{id} . ",\n";
print " \"name\": \"" . $product->{name} . "\",\n";
print " \"price\": " . $product->{price} . "\n";
print " }";
}
print "\n ]\n";
print "}\n";
}
elsif ($action eq 'get' && $id) {
my $sth = $dbh->prepare("SELECT id, name, price, description FROM products WHERE id = ?");
$sth->execute($id);
my $product = $sth->fetchrow_hashref;
if ($product) {
print "{\n";
print " \"id\": " . $product->{id} . ",\n";
print " \"name\": \"" . $product->{name} . "\",\n";
print " \"price\": " . $product->{price} . ",\n";
print " \"description\": \"" . $product->{description} . "\"\n";
print "}\n";
} else {
print "{\n";
print " \"error\": \"Product not found\"\n";
print "}\n";
}
}
$dbh->disconnect();
Early API Authentication Methods
Authentication was typically simple and often insecure:
- API Keys in Query Strings: Easily visible in server logs and browser history
- Basic Authentication: Username/password encoded in Base64
- Custom Authentication Headers: Non-standardized proprietary headers
- Session Cookies: Using the same authentication as web applications
Integration Challenges
Early API integrations were typically one-off, custom developments with brittle connections between systems. Changes to either endpoint often broke the integration, requiring manual updates to maintain functionality.
The SOAP Era: Enterprise Web Services (2000-2010)
The early 2000s saw the rise of SOAP (Simple Object Access Protocol) and associated WS-* standards, bringing formality and structure to API development:
Key Characteristics
- SOAP Protocol: XML-based messaging format
- WSDL: Web Services Description Language
- WS-* Standards: Security, Addressing, etc.
- Enterprise Focus: Strong typing, contracts
- SOA Architecture: Service Oriented Architecture
- Transport Independence: HTTP, SMTP, etc.
API Management Approach
- Formal service contracts
- XML Schema validation
- Enterprise Service Buses (ESBs)
- Sophisticated error handling
- Complex authentication
- Heavyweight integration patterns
<!-- SOAP Request (abbreviated) -->
POST /services/UserService HTTP/1.1
Host: api.example.com
Content-Type: application/soap+xml; charset=UTF-8
SOAPAction: "http://example.com/GetUserDetails"
Content-Length: 547
<?xml version="1.0" encoding="UTF-8"?>
<soap:Envelope
xmlns:soap="http://www.w3.org/2003/05/soap-envelope"
xmlns:ns="http://example.com">
<soap:Header>
<ns:Authentication>
<ns:Username>system</ns:Username>
<ns:Password>manager</ns:Password>
</ns:Authentication>
</soap:Header>
<soap:Body>
<ns:GetUserDetails>
<ns:UserId>123</ns:UserId>
</ns:GetUserDetails>
</soap:Body>
</soap:Envelope>
<!-- SOAP Response (abbreviated) -->
HTTP/1.1 200 OK
Content-Type: application/soap+xml; charset=UTF-8
Content-Length: 872
<?xml version="1.0" encoding="UTF-8"?>
<soap:Envelope
xmlns:soap="http://www.w3.org/2003/05/soap-envelope"
xmlns:ns="http://example.com">
<soap:Body>
<ns:GetUserDetailsResponse>
<ns:User>
<ns:UserId>123</ns:UserId>
<ns:Username>johndoe</ns:Username>
<ns:Email>john@example.com</ns:Email>
<ns:FirstName>John</ns:FirstName>
<ns:LastName>Doe</ns:LastName>
<ns:Address>
<ns:Street>123 Main St</ns:Street>
<ns:City>Anytown</ns:City>
<ns:State>CA</ns:State>
<ns:PostalCode>12345</ns:PostalCode>
</ns:Address>
<ns:PhoneNumbers>
<ns:PhoneNumber type="Home">555-123-4567</ns:PhoneNumber>
<ns:PhoneNumber type="Mobile">555-987-6543</ns:PhoneNumber>
</ns:PhoneNumbers>
</ns:User>
</ns:GetUserDetailsResponse>
</soap:Body>
</soap:Envelope>
<?xml version="1.0" encoding="UTF-8"?>
<definitions
name="UserService"
targetNamespace="http://example.com/wsdl"
xmlns="http://schemas.xmlsoap.org/wsdl/"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:tns="http://example.com/wsdl"
xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<types>
<xsd:schema targetNamespace="http://example.com/wsdl">
<!-- Complex type definitions -->
<xsd:complexType name="User">
<xsd:sequence>
<xsd:element name="UserId" type="xsd:integer"/>
<xsd:element name="Username" type="xsd:string"/>
<xsd:element name="Email" type="xsd:string"/>
<xsd:element name="FirstName" type="xsd:string"/>
<xsd:element name="LastName" type="xsd:string"/>
<!-- Additional elements -->
</xsd:sequence>
</xsd:complexType>
<!-- Request and response message types -->
<xsd:element name="GetUserDetailsRequest">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="UserId" type="xsd:integer"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
<xsd:element name="GetUserDetailsResponse">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="User" type="tns:User"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
</xsd:schema>
</types>
<!-- Message definitions -->
<message name="GetUserDetailsInput">
<part name="parameters" element="tns:GetUserDetailsRequest"/>
</message>
<message name="GetUserDetailsOutput">
<part name="parameters" element="tns:GetUserDetailsResponse"/>
</message>
<!-- Port Type (Interface) -->
<portType name="UserServicePortType">
<operation name="GetUserDetails">
<input message="tns:GetUserDetailsInput"/>
<output message="tns:GetUserDetailsOutput"/>
</operation>
<!-- Other operations -->
</portType>
<!-- Binding to SOAP protocol -->
<binding name="UserServiceSOAP" type="tns:UserServicePortType">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="GetUserDetails">
<soap:operation soapAction="http://example.com/GetUserDetails"/>
<input><soap:body use="literal"/></input>
<output><soap:body use="literal"/></output>
</operation>
</binding>
<!-- Service definition -->
<service name="UserService">
<port name="UserServicePort" binding="tns:UserServiceSOAP">
<soap:address location="http://api.example.com/services/UserService"/>
</port>
</service>
</definitions>
Enterprise Authentication Patterns
SOAP APIs introduced more sophisticated authentication mechanisms:
- WS-Security: XML-based security tokens
- SAML: Security Assertion Markup Language for federated authentication
- X.509 Certificates: Client certificate authentication
- Custom SOAP Headers: Proprietary authentication tokens
The Complexity Problem
While SOAP provided robust enterprise features, its complexity made it challenging to implement and maintain. Developers needed specialized tools and training, and the heavy XML payloads were inefficient for many web applications, especially as mobile usage increased.
The REST Revolution: Simplicity and Resources (2005-2015)
REST (Representational State Transfer) emerged as a simpler alternative to SOAP, focusing on resources and standard HTTP methods:
Key REST Principles
- Resource-Based: Everything is a resource with a URI
- Standard HTTP Methods: GET, POST, PUT, DELETE
- Stateless: No client context stored on server
- Uniform Interface: Consistent resource interaction
- Hypermedia (HATEOAS): Links between resources
- Multiple Representations: JSON, XML, etc.
REST Advantages
- Simpler implementation than SOAP
- Lightweight JSON payloads
- Easier integration for web and mobile apps
- Better performance and scalability
- Leveraged existing HTTP infrastructure
- Developer-friendly approach
GET /api/users/123 HTTP/1.1
Host: api.example.com
Accept: application/json
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
HTTP/1.1 200 OK
Content-Type: application/json
Cache-Control: max-age=3600
{
"id": 123,
"username": "johndoe",
"email": "john@example.com",
"firstName": "John",
"lastName": "Doe",
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"postalCode": "12345"
},
"phoneNumbers": [
{ "type": "home", "number": "555-123-4567" },
{ "type": "mobile", "number": "555-987-6543" }
],
"_links": {
"self": { "href": "/api/users/123" },
"orders": { "href": "/api/users/123/orders" },
"profile": { "href": "/api/users/123/profile" }
}
}
# Create a new user
POST /api/users HTTP/1.1
Host: api.example.com
Content-Type: application/json
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
{
"username": "newuser",
"email": "new@example.com",
"firstName": "New",
"lastName": "User"
}
# Read a specific user
GET /api/users/123 HTTP/1.1
Host: api.example.com
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
# Update a user
PUT /api/users/123 HTTP/1.1
Host: api.example.com
Content-Type: application/json
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
{
"email": "updated@example.com",
"firstName": "Updated"
}
# Delete a user
DELETE /api/users/123 HTTP/1.1
Host: api.example.com
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
API Authentication Diversification
The REST era saw a proliferation of authentication methods:
Authentication Method | Implementation Approach | Common Use Cases | Advantages | Disadvantages |
---|---|---|---|---|
API Keys | Secret key in header or parameter | Public APIs, Developer SDKs | Simple implementation | Limited security, no expiration |
OAuth 1.0 | Cryptographic signature workflow | Twitter API (originally) | Secure without HTTPS | Complex implementation |
OAuth 2.0 | Token-based delegated access | Social login, third-party access | Delegated permissions | Multiple flows to implement |
JWT (JSON Web Tokens) | Self-contained signed tokens | Microservices, SPAs | Stateless, contains claims | Token size, revocation challenges |
HMAC Signatures | Cryptographic request signing | AWS, financial APIs | Strong security | Implementation complexity |
The Rise of API Description Formats
As REST APIs became more common, various description formats emerged to document them:
- Swagger/OpenAPI: Machine-readable API specifications
- RAML: RESTful API Modeling Language
- API Blueprint: Markdown-based API descriptions
- WADL: Web Application Description Language (XML-based)
openapi: 3.0.0
info:
title: User API
description: API for managing users
version: 1.0.0
servers:
- url: https://api.example.com
paths:
/users:
get:
summary: Returns a list of users
parameters:
- name: limit
in: query
schema:
type: integer
default: 20
- name: offset
in: query
schema:
type: integer
default: 0
responses:
'200':
description: A JSON array of user objects
content:
application/json:
schema:
type: object
properties:
data:
type: array
items:
$ref: '#/components/schemas/User'
pagination:
$ref: '#/components/schemas/Pagination'
post:
summary: Creates a new user
requestBody:
required: true
content:
application/json:
schema:
$ref: '#/components/schemas/NewUser'
responses:
'201':
description: Created
content:
application/json:
schema:
$ref: '#/components/schemas/User'
'400':
description: Bad request
/users/{id}:
get:
summary: Returns a user by ID
parameters:
- name: id
in: path
required: true
schema:
type: integer
responses:
'200':
description: A user object
content:
application/json:
schema:
$ref: '#/components/schemas/User'
'404':
description: User not found
components:
schemas:
User:
type: object
properties:
id:
type: integer
username:
type: string
email:
type: string
firstName:
type: string
lastName:
type: string
# Additional properties...
required:
- id
- username
- email
NewUser:
type: object
properties:
username:
type: string
email:
type: string
firstName:
type: string
lastName:
type: string
required:
- username
- email
Pagination:
type: object
properties:
total:
type: integer
limit:
type: integer
offset:
type: integer
nextPage:
type: string
format: uri
prevPage:
type: string
format: uri
securitySchemes:
bearerAuth:
type: http
scheme: bearer
bearerFormat: JWT
security:
- bearerAuth: []
The HATEOAS Challenge
One of the most frequently ignored aspects of REST was HATEOAS (Hypermedia as the Engine of Application State). While theoretically elegant, enabling clients to navigate APIs through embedded links, it proved complex to implement and consume in practice. Many "RESTful" APIs adopted a pragmatic subset of REST principles, leading to what some called "REST-ish" APIs.
API Management and Monetization (2010-Present)
As APIs became business assets, specialized management platforms emerged to handle security, analytics, and monetization:
Key Capabilities
- Developer Portals: Self-service API access
- API Gateways: Security and traffic management
- Usage Analytics: Monitoring and reporting
- Rate Limiting: Controlling consumption
- Versioning: Managing API lifecycle
- Monetization: Billing and payment processing
Common API Business Models
- Freemium: Free tier with paid upgrades
- Tiered Pricing: Different feature/usage levels
- Pay-as-you-go: Usage-based billing
- Subscription: Recurring access fees
- Transaction Fees: Per-transaction charges
- Revenue Sharing: Splitting revenue with partners
The API Billing Model Fragmentation
API providers implemented wildly different billing structures, creating complexity for consumers:
API Provider Example | Billing Model | Metering Approach | Cost Structure |
---|---|---|---|
Twilio | Per-message/call pricing | Counts each API interaction | Different prices by country/service |
Stripe | Percentage of transaction | Based on payment volume | % + fixed fee per transaction |
Google Maps | Credit-based with monthly free tier | Different credits for different operations | SKU-based pricing by feature |
AWS | Resource consumption with tiers | Multiple dimensions (requests, data, compute) | Complex matrix pricing with volume discounts |
GitHub | Monthly subscription | Unlimited API access within rate limits | Flat fee based on user/organization plan |
API Specifications and Documentation
Documentation became a critical differentiator for API providers:
Tools like Swagger UI and Postman allowed developers to explore and test APIs directly from documentation.
This radical improvement in developer experience became a standard expectation for quality APIs.
Auto-generated client libraries in multiple languages reduced integration friction.
Example code for common operations became standard in API documentation.
Specifications became design artifacts, created before implementation.
This enabled parallel work on client and server implementations based on a shared contract.
The API Integration Burden
The proliferation of APIs with different authentication methods, data formats, error handling approaches, and billing models created significant overhead for developers integrating multiple services. A typical web application might need to integrate payment processing, mapping, authentication, email delivery, and analytics APIs, each with their own unique integration patterns and billing structures.
GraphQL: Client-Driven APIs (2015-Present)
GraphQL emerged from Facebook as a new approach to API design, focusing on client data needs rather than server resources:
Key GraphQL Features
- Client-Specified Queries: Request exactly what you need
- Single Endpoint: One URL for all operations
- Strong Type System: Schema-defined API
- Hierarchical Data: Natural resolution of nested data
- Introspection: Self-documenting APIs
- Real-time Support: Subscriptions for live data
GraphQL Advantages
- Reduced network overhead
- No under/over-fetching of data
- Parallel resolution of fields
- Strong typing prevents errors
- Versioning not required
- Single request for complex data
# GraphQL Query
query GetUserWithOrders {
user(id: 123) {
id
username
email
firstName
lastName
address {
street
city
state
postalCode
}
orders(last: 3) {
id
orderDate
status
total
items {
product {
id
name
price
}
quantity
subtotal
}
}
}
}
# Response
{
"data": {
"user": {
"id": "123",
"username": "johndoe",
"email": "john@example.com",
"firstName": "John",
"lastName": "Doe",
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"postalCode": "12345"
},
"orders": [
{
"id": "1001",
"orderDate": "2025-05-01",
"status": "DELIVERED",
"total": 148.95,
"items": [
{
"product": {
"id": "p123",
"name": "Wireless Headphones",
"price": 99.95
},
"quantity": 1,
"subtotal": 99.95
},
{
"product": {
"id": "p456",
"name": "Phone Case",
"price": 24.50
},
"quantity": 2,
"subtotal": 49.00
}
]
},
{
"id": "982",
"orderDate": "2025-04-15",
"status": "DELIVERED",
"total": 39.99,
"items": [
{
"product": {
"id": "p789",
"name": "T-Shirt",
"price": 19.99
},
"quantity": 2,
"subtotal": 39.98
}
]
}
]
}
}
}
# GraphQL Schema
type User {
id: ID!
username: String!
email: String!
firstName: String
lastName: String
address: Address
phoneNumbers: [PhoneNumber]
orders(last: Int): [Order]
}
type Address {
street: String
city: String
state: String
postalCode: String
country: String
}
type PhoneNumber {
type: PhoneType
number: String!
}
enum PhoneType {
HOME
WORK
MOBILE
}
type Order {
id: ID!
orderDate: String!
status: OrderStatus!
total: Float!
items: [OrderItem!]!
}
enum OrderStatus {
PENDING
PROCESSING
SHIPPED
DELIVERED
CANCELLED
}
type OrderItem {
product: Product!
quantity: Int!
subtotal: Float!
}
type Product {
id: ID!
name: String!
description: String
price: Float!
category: Category
inStock: Boolean!
}
type Category {
id: ID!
name: String!
products: [Product]
}
# Query entry points
type Query {
user(id: ID!): User
users(limit: Int, offset: Int): [User]
product(id: ID!): Product
products(category: ID, search: String, limit: Int, offset: Int): [Product]
order(id: ID!): Order
}
# Mutations
type Mutation {
createUser(input: UserInput!): User
updateUser(id: ID!, input: UserInput!): User
deleteUser(id: ID!): Boolean
createOrder(input: OrderInput!): Order
}
input UserInput {
username: String
email: String
firstName: String
lastName: String
address: AddressInput
}
input AddressInput {
street: String
city: String
state: String
postalCode: String
country: String
}
input OrderInput {
userId: ID!
items: [OrderItemInput!]!
}
input OrderItemInput {
productId: ID!
quantity: Int!
}
# Subscriptions for real-time updates
type Subscription {
orderStatusChanged(orderId: ID!): Order
newProductAnnouncement: Product
}
GraphQL Authentication Approaches
GraphQL doesn't specify an authentication method, leading to various implementation patterns:
- HTTP Headers: Using standard Authorization headers
- Context Providers: Framework-specific auth middleware
- Apollo Federation: Service-to-service authentication
- Directives: Schema-level access control
- Centralized vs. Per-Resolver Auth: Different granularity levels
// Apollo Server with authentication
const { ApolloServer, gql } = require('apollo-server-express');
const jwt = require('jsonwebtoken');
// Schema definition with auth directives
const typeDefs = gql`
directive @auth(requires: Role = USER) on OBJECT | FIELD_DEFINITION
enum Role {
USER
ADMIN
}
type User {
id: ID!
username: String!
email: String! @auth(requires: ADMIN)
orders: [Order!]!
}
type Order @auth(requires: USER) {
id: ID!
items: [OrderItem!]!
total: Float!
}
# Other type definitions...
type Query {
me: User @auth
users: [User!]! @auth(requires: ADMIN)
}
`;
// Resolver functions
const resolvers = {
Query: {
me: (_, __, context) => {
// User must be authenticated to access this
if (!context.user) {
throw new Error('Not authenticated');
}
return context.dataSources.users.findById(context.user.id);
},
users: (_, __, context) => {
// Only admin users can access this
if (!context.user || context.user.role !== 'ADMIN') {
throw new Error('Not authorized');
}
return context.dataSources.users.findAll();
}
}
};
// Auth directive implementation
const AuthDirective = require('./directives/auth');
// Create Apollo Server
const server = new ApolloServer({
typeDefs,
resolvers,
schemaDirectives: {
auth: AuthDirective
},
context: ({ req }) => {
// Extract and verify JWT from Authorization header
const token = req.headers.authorization || '';
if (token) {
try {
const user = jwt.verify(token.replace('Bearer ', ''), process.env.JWT_SECRET);
return { user };
} catch (error) {
console.error('Invalid token:', error.message);
}
}
// Return empty context if not authenticated
return { user: null };
}
});
The API Size/Scope Tradeoff
GraphQL introduced a different API design tension: Should you create one large unified graph (as Facebook does) or multiple smaller, domain-specific GraphQL APIs? Large graphs provide maximum flexibility to clients but create complex dependency and permission management challenges. This tension continues to shape API architecture decisions today.
Modern API Ecosystem & Challenges (2020-Present)
Today's API landscape features unprecedented diversity in approaches, blending different paradigms to meet specific needs:
Current API Approaches
- REST APIs: Still the most common approach
- GraphQL: Growing adoption for flexible data needs
- gRPC: High-performance service-to-service communication
- WebSockets: Real-time bidirectional communication
- Webhooks: Event-driven integrations
- Serverless Functions: Event-triggered micro-APIs
- BFFs (Backend for Frontend): Client-specific API layers
Persistent Challenges
- API Discovery: Finding available APIs
- Heterogeneous Ecosystems: Different patterns across APIs
- Authentication Complexity: Multiple auth schemes
- Versioning Strategies: Handling API evolution
- Rate Limiting Variability: Different throttling approaches
- Documentation Quality: Inconsistent standards
- Error Handling: Non-standardized error formats
API Federation and Gateway Patterns
To manage API complexity, various federation patterns have emerged:
# Kong API Gateway configuration example
_format_version: "2.1"
services:
- name: user-service
url: http://user-service:8080
routes:
- name: user-api
paths:
- /api/users
strip_path: false
plugins:
- name: rate-limiting
config:
minute: 60
policy: local
- name: key-auth
config:
key_names: [api_key]
- name: cors
config:
origins:
- '*'
methods:
- GET
- POST
- PUT
- DELETE
headers:
- Accept
- Content-Type
- Authorization
max_age: 3600
- name: order-service
url: http://order-service:8080
routes:
- name: order-api
paths:
- /api/orders
strip_path: false
plugins:
- name: jwt
config:
claims_to_verify:
- exp
key_claim_name: kid
secret_is_base64: false
- name: acl
config:
allow:
- orders-group
- name: request-transformer
config:
add:
headers:
- X-Consumer-ID:$(consumer.id)
- X-Consumer-Username:$(consumer.username)
- name: product-service
url: http://product-service:8080
routes:
- name: product-api
paths:
- /api/products
strip_path: false
plugins:
- name: oauth2
config:
enable_client_credentials: true
enable_authorization_code: true
mandatory_scope: true
provision_key: "PROVISION_KEY"
token_expiration: 7200
scopes:
- "products:read"
- "products:write"
- name: rate-limiting
config:
minute: 120
policy: redis
redis_host: redis
redis_port: 6379
consumers:
- username: mobile-app
keyauth_credentials:
- key: MOBILE_API_KEY
acls:
- group: orders-group
- username: partner-service
jwt_secrets:
- key: "PARTNER_SECRET_KEY"
acls:
- group: orders-group
- username: admin-dashboard
oauth2_credentials:
- name: "Admin Dashboard"
client_id: "ADMIN_CLIENT_ID"
client_secret: "ADMIN_CLIENT_SECRET"
redirect_uris:
- https://admin.example.com/oauth/callback
The Persistent Authentication Problem
Authentication remains a significant challenge, with multiple patterns across the ecosystem:
API Provider Example | Authentication Method | Credential Storage | Token Lifespan | Implementation Quirks |
---|---|---|---|---|
Stripe | API Key (Bearer Token) | Dashboard-generated secret key | Non-expiring, revocable | Test/live key pairs, restricted keys |
OAuth 2.0 + API Key | Google Cloud Console | Access token: 1hr Refresh token: long-lived |
Service account JSON keys, API key restrictions | |
GitHub | OAuth Apps + Personal Access Tokens | GitHub settings | Token: configurable expiry | Fine-grained permissions, OAuth device flow |
AWS | HMAC Signature (v4 Signing) | IAM access/secret keys | Signature valid for 15 min | Complex signing process, temporary credentials |
Salesforce | OAuth 2.0 JWT Bearer Flow | Connected App configuration | Session-based, configurable | Multiple domains, my domain considerations |
Webhook Standardization Efforts
Webhooks have emerged as a critical API pattern for event-driven architectures, yet implementation varies widely:
{
"specversion": "1.0",
"type": "com.example.order.created",
"source": "/orders/api",
"id": "1234-5678-9101",
"time": "2025-05-15T17:31:00Z",
"datacontenttype": "application/json",
"data": {
"orderId": "ord_123456",
"customer": {
"id": "cust_987654",
"email": "customer@example.com"
},
"items": [
{
"productId": "prod_abc123",
"quantity": 2,
"price": 29.99
}
],
"total": 59.98,
"status": "pending"
}
}
The API Cost Management Problem
With different pricing models across APIs, cost management has become a specialized discipline:
- API Usage Monitoring: Tracking consumption across services
- Cost Allocation: Attributing API costs to business functions
- Request Optimization: Batching, caching to reduce costs
- Rate Limit Management: Avoiding overage charges
- Multi-account Strategies: Separating development/production usage
- Usage-Based Pricing: Passing costs to end-users
The API Integration Bottleneck
Despite dramatic improvements in API technologies, integration remains a bottleneck for many organizations. The heterogeneous landscape of authentication methods, data formats, error handling, and pricing models creates significant cognitive load for developers. Each new API integration requires learning service-specific patterns and quirks, slowing down development velocity.
The Future of API Support in Web Frameworks
Looking ahead, several trends are shaping how web frameworks approach API integration:
Frameworks are beginning to offer higher-level abstractions that normalize differences between third-party APIs.
This includes unified authentication handling, error normalization, and consistent request/response patterns across diverse services.
Examples include Commerce.js, Contentful's App Framework, and payment abstraction libraries.
Automatic code generation from OpenAPI and GraphQL schemas is becoming standard in modern frameworks.
This removes boilerplate, ensures type safety, and handles many edge cases automatically.
Examples include Apollo Codegen, OpenAPI Generator, and tRPC's type inference.
Frameworks are providing seamless API proxying through edge functions, solving CORS issues and enabling credential hiding.
This approach also enables request/response transformation at the edge for API normalization.
Examples include Netlify Functions, Vercel Edge Middleware, and Cloudflare Workers.
AI-Assisted API Integration
AI-powered tools are emerging to simplify API integration:
- Automatic Documentation Parsing: AI understanding API usage from docs
- Code Generation: Creating integration code from natural language
- Error Diagnosis: Explaining API errors and suggesting solutions
- Data Transformation: Automatically mapping between different schemas
- API Discovery: Finding appropriate APIs for specific tasks
New API Paradigms on the Horizon
Emerging approaches that may shape future API integration:
- Streaming APIs: Push-based data flows with backpressure
- WASI: WebAssembly System Interface for portable APIs
- Functional Data APIs: Expressing data needs as composable functions
- AI-mediated APIs: Natural language interfaces to services
- Protocol Buffers/FlatBuffers: Efficient binary protocols for edge computing
// Modern framework approach to API integration
// app/api/[[...slug]]/route.ts
import { NextRequest, NextResponse } from 'next/server';
import { headers } from 'next/headers';
import { getToken } from 'next-auth/jwt';
// API service configuration
const API_SERVICES = {
payments: {
baseUrl: process.env.PAYMENT_API_URL,
authType: 'bearer',
secret: process.env.PAYMENT_API_SECRET,
},
products: {
baseUrl: process.env.PRODUCT_API_URL,
authType: 'apiKey',
headerName: 'X-API-Key',
secret: process.env.PRODUCT_API_KEY,
},
users: {
baseUrl: process.env.USERS_API_URL,
authType: 'oauth',
clientId: process.env.OAUTH_CLIENT_ID,
clientSecret: process.env.OAUTH_CLIENT_SECRET,
}
};
// Rate limiting configuration
const RATE_LIMITS = {
anonymous: 20, // per minute
authenticated: 100, // per minute
};
export async function GET(request: NextRequest, { params }: { params: { slug?: string[] } }) {
return handleApiRequest(request, params, 'GET');
}
export async function POST(request: NextRequest, { params }: { params: { slug?: string[] } }) {
return handleApiRequest(request, params, 'POST');
}
export async function PUT(request: NextRequest, { params }: { params: { slug?: string[] } }) {
return handleApiRequest(request, params, 'PUT');
}
export async function DELETE(request: NextRequest, { params }: { params: { slug?: string[] } }) {
return handleApiRequest(request, params, 'DELETE');
}
async function handleApiRequest(
request: NextRequest,
params: { slug?: string[] },
method: string
) {
// Rate limiting
const token = await getToken({ req: request as any });
const isAuthenticated = !!token;
const rateLimit = isAuthenticated ? RATE_LIMITS.authenticated : RATE_LIMITS.anonymous;
// Extract service and path
const [service, ...pathParts] = params.slug || [];
const path = pathParts.join('/');
if (!service || !API_SERVICES[service]) {
return NextResponse.json(
{ error: 'Invalid API service specified' },
{ status: 400 }
);
}
const serviceConfig = API_SERVICES[service];
const url = `${serviceConfig.baseUrl}/${path}${request.nextUrl.search}`;
// Prepare headers
const requestHeaders = new Headers(headers() as HeadersInit);
requestHeaders.delete('host');
// Add authentication based on service config
if (serviceConfig.authType === 'bearer') {
requestHeaders.set('Authorization', `Bearer ${serviceConfig.secret}`);
} else if (serviceConfig.authType === 'apiKey') {
requestHeaders.set(serviceConfig.headerName, serviceConfig.secret);
} else if (serviceConfig.authType === 'oauth') {
// Fetch OAuth token (simplified)
const tokenResponse = await fetch(`${serviceConfig.baseUrl}/oauth/token`, {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: new URLSearchParams({
grant_type: 'client_credentials',
client_id: serviceConfig.clientId,
client_secret: serviceConfig.clientSecret,
}),
});
const { access_token } = await tokenResponse.json();
requestHeaders.set('Authorization', `Bearer ${access_token}`);
}
// Forward the request to the target API
const options: RequestInit = {
method,
headers: requestHeaders,
};
// Include body for methods that support it
if (['POST', 'PUT', 'PATCH'].includes(method)) {
const body = await request.json().catch(() => ({}));
options.body = JSON.stringify(body);
}
try {
const response = await fetch(url, options);
// Transform the response
const data = await response.json();
// Normalize error responses
if (!response.ok) {
// Log API error details
console.error(`API ${service} error:`, data);
// Return a normalized error response
return NextResponse.json(
{
error: true,
message: data.message || data.error || 'An error occurred',
code: data.code || response.status.toString(),
service
},
{ status: response.status }
);
}
// Return successful response
return NextResponse.json(data, {
status: response.status,
headers: {
'Cache-Control': response.headers.get('Cache-Control') || 'no-cache',
},
});
} catch (error) {
console.error(`Error calling ${service} API:`, error);
return NextResponse.json(
{ error: 'Service unavailable', service },
{ status: 503 }
);
}
}
The Event-Driven Future
As applications become more real-time and interconnected, event-driven architecture is becoming central to API design. The combination of webhooks, WebSockets, and event streaming platforms is creating a more reactive approach to integration. Future frameworks will likely provide first-class support for these patterns, including standardized event schemas, automatic retry mechanisms, and comprehensive event monitoring.
Conclusion
APIs have evolved from simple CGI scripts to sophisticated GraphQL schemas, reflecting the increasing importance of software integration in modern applications. Throughout this evolution, we've seen pendulum swings between simplicity and power, standardization and specialization.
The diversity of API standards, authentication methods, data formats, and billing models remains a significant challenge for developers. While each approach offers advantages for specific use cases, the lack of standardization creates friction in the integration process.
Modern web frameworks are beginning to address these challenges through higher-level abstractions, code generation, and edge-enabled proxies. However, a truly universal solution to API integration complexity remains elusive.
As we look to the future, the successful approach may not be a single unified standard, but rather improved tooling that abstracts away the differences between APIs, allowing developers to focus on functionality rather than integration details. The combination of AI-assisted development, standardized gateway patterns, and improved framework support promises to reduce the API integration burden and accelerate web application development.